امکان دسترسی به محتوای GridView با استفاده از رویداد RowDataBound و RowCommand
پنجشنبه 20 فروردین 1394در این مقاله نحوه دسترسی و نمایش محتوای داخل کنترل GridView را با استفاده از رویداد RowCommand و RowDataBound در #C و VB.Net توضیح خواهیم داد
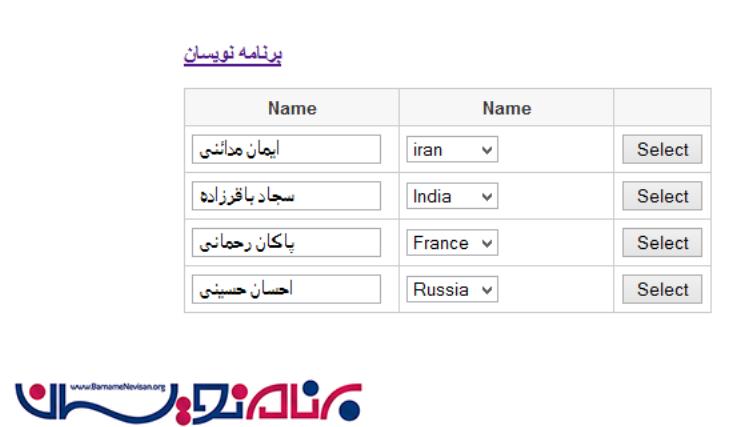
برای نمایش محتوای گرید ویو مطابق مراحل زیر عمل میکنیم :
ابتدا یک کنترل GridView که شامل دو TemplateField به صفحه اضافه می کنیم .همچنین کنترل GridView شامل Command Button و رویدادهای OnRowDataBound و OnRowCommand میباشد .
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" OnRowDataBound="GridView1_RowDataBound" OnRowCommand="GridView1_RowCommand"> <Columns> <asp:TemplateField HeaderText="Name" ItemStyle-Width="150"> <ItemTemplate> <asp:TextBox ID="txtName" runat="server" Text='<%# Eval("Name") %>' /> </ItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="Name" ItemStyle-Width="150"> <ItemTemplate> <asp:DropDownList ID="ddlCountries" runat="server"> <asp:ListItem Text="United States" Value="United States" /> <asp:ListItem Text="India" Value="India" /> <asp:ListItem Text="France" Value="France" /> <asp:ListItem Text="Russia" Value="Russia" /> </asp:DropDownList> </ItemTemplate> </asp:TemplateField> <asp:ButtonField CommandName="Select" Text="Select" ButtonType="Button" /> </Columns> </asp:GridView>
سپس فضای نامهای زیر را اظافه میکنیم :
کد #C:
using System.Data;
کد VB.Net :
Imports System.Data
سپس یک DataTable ایجاد میکنیم و کنترل GridView را به آن متصل می کنیم :
کد #C :
protected void Page_Load(object sender, EventArgs e) { if (!this.IsPostBack) { DataTable dt = new DataTable(); dt.Columns.AddRange(new DataColumn[3] { new DataColumn("Id"), new DataColumn("Name"), new DataColumn("Country") }); dt.Rows.Add(1, " ایمان مدائنی ", "iran"); dt.Rows.Add(2, " سجاد باقرزاده", "India"); dt.Rows.Add(3, " پاکان رحمانی", "France"); dt.Rows.Add(4, " احسان حسینی", "Russia"); GridView1.DataSource = dt; GridView1.DataBind(); } }
کد VB.Net :
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load If Not Me.IsPostBack Then Dim dt As New DataTable() dt.Columns.AddRange(New DataColumn(2) {New DataColumn("Id"), New DataColumn("Name"), New DataColumn("Country")}) dt.Rows.Add(1, "John Hammond", "United States") dt.Rows.Add(2, "Mudassar Khan", "India") dt.Rows.Add(3, "Suzanne Mathews", "France") dt.Rows.Add(4, "Robert Schidner", "Russia") GridView1.DataSource = dt GridView1.DataBind() End If End Sub
در داخل رویداد RowDataBound ابتدا از اتصال GidView به DataTable اطمینان حاصل میشود , سپس محتوای داخل TextBox و DropDownList از طریق ID آن سطر تشخیص داده می شود و در ادامه محتوای آناها به پارامتر مورد نظر ارسال می گردد .
کد #C :
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e) { if (e.Row.RowType == DataControlRowType.DataRow) { //Find the TextBox control. TextBox txtName = (e.Row.FindControl("txtName") as TextBox); //Find the DropDownList control. DropDownList ddlCountries = (e.Row.FindControl("ddlCountries") as DropDownList); string country = (e.Row.DataItem as DataRowView)["Country"].ToString(); ddlCountries.Items.FindByValue(country).Selected = true; } }
کد VB.Net :
Protected Sub GridView1_RowDataBound(sender As Object, e As GridViewRowEventArgs) If e.Row.RowType = DataControlRowType.DataRow Then 'Find the TextBox control. Dim txtName As TextBox = TryCast(e.Row.FindControl("txtName"), TextBox) 'Find the DropDownList control. Dim ddlCountries As DropDownList = TryCast(e.Row.FindControl("ddlCountries"), DropDownList) Dim country As String = TryCast(e.Row.DataItem, DataRowView)("Country").ToString() ddlCountries.Items.FindByValue(country).Selected = True End If End Sub
تصویر زیر نحوه اتصال کنترل به رویداد RowDataBound را نمایش میدهد .
مقدار وارد شده در سطر به راحتی با خاصیت CommandArgument قابل تشخیص میباشد .سپس مقدار داخل سطر انتخاب شده از طریق دستور JavaScript به کاربر نمایش داده میشود .
کد #C :
protected void GridView1_RowCommand(object sender, GridViewCommandEventArgs e) { //Determine the RowIndex of the Row whose Button was clicked. int rowIndex = Convert.ToInt32(e.CommandArgument); //Reference the GridView Row. GridViewRow row = GridView1.Rows[rowIndex]; //Find the TextBox control. TextBox txtName = (row.FindControl("txtName") as TextBox); //Find the DropDownList control. DropDownList ddlCountries = (row.FindControl("ddlCountries") as DropDownList); ClientScript.RegisterStartupScript(this.GetType(), "alert", "alert('Name: " + txtName.Text + "\\nCountry: " + ddlCountries.SelectedItem.Value + "');", true); }
کد Vb.Net:
Protected Sub GridView1_RowCommand(sender As Object, e As GridViewCommandEventArgs) 'Determine the RowIndex of the Row whose Button was clicked. Dim rowIndex As Integer = Convert.ToInt32(e.CommandArgument) 'Reference the GridView Row. Dim row As GridViewRow = GridView1.Rows(rowIndex) 'Find the TextBox control. Dim txtName As TextBox = TryCast(row.FindControl("txtName"), TextBox) 'Find the DropDownList control. Dim ddlCountries As DropDownList = TryCast(row.FindControl("ddlCountries"), DropDownList) ClientScript.RegisterStartupScript(Me.[GetType](), "alert", "alert('Name: " + txtName.Text + "\nCountry: " + ddlCountries.SelectedItem.Value + "');", True) End Sub
- ASP.net
- 4k بازدید
- 2 تشکر