بازگشت داده به فرمت JSON در MVC
یکشنبه 20 اردیبهشت 1394در اینجا بازگشت داده به فرمت JSON در MVC را شرح میدهیم.
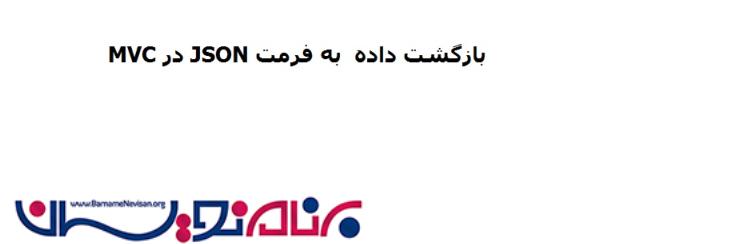
JSON : JavaScript Object Notation :
json یک فرمت تبادل داده سبک وزن میباشد که برای انتقال داده بین پلت فرم ها استفاده میشود.
یک کنترلر به نام Members میسازیم و دو مدل regidtration و connection
یک جدول به شکل زیر داریم:
میخواهیم این داده را با json در mvc انتقال دهیم ابتدا در کنترلر member کد زیر را بنویسید:
using System.Data.SqlClient; using MVCPROJECT.Models; namespace MVCPROJECT.Controllers { public class MembersController: Controller { List < Dictionary < string, object >> rows = new List < Dictionary < string, object >> (); //creating a list to hold the rows of datatable Dictionary < string, object > rowelement; //Initialise a dictionary because it will contain columnName and Column Value and the key is column Name [HttpGet public JsonResult Show() { Registration obj = new Registration(); //Model object is created DataTable dt = new DataTable(); dt = obj.employeedetails(); //calling a method which declared in model to retrive all data from the table and store it in dt. if (dt.Rows.Count > 0) //if data is there in dt(dataTable) { foreach(DataRow dr in dt.Rows) { rowelement = new Dictionary < string, object > (); foreach(DataColumn col in dt.Columns) { rowelement.Add(col.ColumnName, dr[col]); //adding columnn } rows.Add(rowelement); } } return Json(rows, JsonRequestBehavior.AllowGet); } } }
در پوشه مدل خود دو مدل به نام های employee و connection داریم در کلاس employee خصوصیات پایگاه داده با متد employeedetail :
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Data; using System.Data.SqlClient; using MVCPROJECT.Models; using Forms.Models; namespace MVCPROJECT.Models { public class Registration { Connection objConnection = new Connection();#region "Properties" SqlDataAdapter ds = new SqlDataAdapter(); DataTable dt = new DataTable(); public string Fname {get;set;} public string SName {get;set;} public string Lname {get;set;} public string Id {get;set;} public string EmailId {get;set;} public string Password {get;set;} #endregion public DataTable employeedetails() { DataTable dt = new DataTable(); dt = objConnection.GetDataTable1("sp_selectemployee"); //GetDataTable1 is my method in connection.class return dt; } } }
و در کلاس Connection برای اتصال به پایگاه داده استفاده میکنیم :
using System; using System.Collections; using System.Configuration; using System.Data; using System.Data.SqlClient; using Forms.Models; namespace Forms.Models { public class Connection { public SqlCommand cmdData = new SqlCommand(); public SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings[1].ConnectionString); public SqlDataAdapter dataAdapter = new SqlDataAdapter(); DataTable dt = new DataTable(); private string connStr = ConfigurationManager.ConnectionStrings["connect"].ConnectionString; public void ExecuteCommand(string procName, CommandType cmdType, Hashtable parameters) { SqlConnection sqlConn = null; try { sqlConn = GetSqlConnection(); if (sqlConn.State == ConnectionState.Closed) { sqlConn.Open(); } SqlCommand sqlComm = GetSqlCommand(procName, cmdType, parameters, sqlConn); sqlComm.ExecuteNonQuery(); } catch (Exception ex) { throw ex; } finally { sqlConn.Close(); } } public DataTable GetDataTable1(string strProcName) { cmdData = new SqlCommand(strProcName); cmdData.CommandType = CommandType.StoredProcedure; cmdData.Connection = conn; dataAdapter = new SqlDataAdapter(cmdData); dt = new DataTable(); dataAdapter.Fill(dt); return dt; } } }
یک procedure در پایگاه داده به شکل زیر مینویسیم :
CREATE procedure [dbo].[sp_selectemployee] ( @mode nvarchar(10)= null, @id nvarchar(10)= null ) as begin select * from tbl_registration end
آدرس را در مرورگر مینویسیم :
/Members/Show
- ASP.net MVC
- 2k بازدید
- 0 تشکر