نحوه ایجاد یک GridView به صورت Modal Pop-Up بر روی یک Gridview دیگر
یکشنبه 7 تیر 1394در این مقاله خواهیم آموخت که چگونه می توانیم یک GridView به صورت Modal Pop-Up بر روی یک Gridview دیگر ایجاد کنیم.
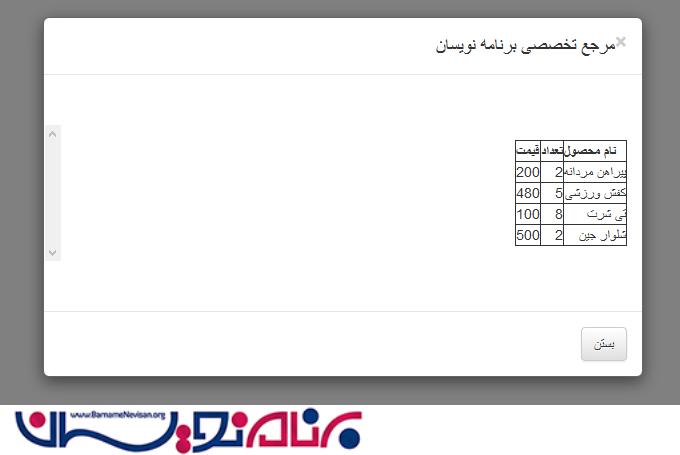
در این مقاله نشان میدهیم که با استفاده از bootstrap چگونه می توانیم یک GridView به صورت Modal Pop-Up بر روی یک Gridview دیگر با کلیک برروی link button نمایش دهیم.
خواهیم آموخت که یک صفحه pop-up بعد از کلیک برروی link button که در کنار اولین Grid قرار دارد نمایش دهیم. صفحه Grid دوم در یک modal-popup از bootstrap باز می شود. Bootstrap برای طراحی صفحات واکنش گرای سایت بسیار مناسب است. در اینجا از کتابخانه jQuery و Bootstrap استفاده کرده ایم.
در صفحه Design در بخش head کتابخانه موردنظر را اضافه کنید. همچنین می توانید این کتابخانه را در کد خود دانلود کرده و به پروژه اضافه کنید.
<script src="http://code.jquery.com/jquery-1.10.2.js"></script> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css"> <!-- Optional theme --> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap-theme.min.css"> <!-- Latest compiled and minified JavaScript --> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/js/bootstrap.min.js"></script>
صفحه موردنظر را با توجه به کدهای زیر طراحی کنید.
<body dir="rtl" > <form id="form1" runat="server"> <div> <asp:GridView ID="GridView1" AutoGenerateColumns="false" OnRowDataBound="GridView1_RowDataBound" runat="server"> <Columns> <asp:BoundField DataField="Product_Name" HeaderText="نام محصول" /> <asp:BoundField DataField="Quantity" HeaderText="تعداد" /> <asp:BoundField DataField="Price" HeaderText="قیمت" /> <asp:TemplateField HeaderText="نمایش جزئیات"> <ItemTemplate> <asp:LinkButton ID="lnkdelete" href="#myModal" data-toggle="modal" runat="server">نمایش اطلاعات</asp:LinkButton> </ItemTemplate> </asp:TemplateField> </Columns> </asp:GridView> </div> <!-- کدهای مربوط به bootstrp modal popup --> <div id="myModal" class="modal fade"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button> <h4 class="modal-title">مرجع تخصصی برنامه نویسان</h4> </div> <div class="modal-body" style="overflow-y: scroll; max-height: 85%; margin-top: 50px; margin-bottom: 50px;"> <asp:Label ID="lblmessage" runat="server" ClientIDMode="Static"></asp:Label> <asp:GridView ID="GridView2" runat="server" AutoGenerateColumns="False"> <Columns> <asp:BoundField DataField="Product_Name" HeaderText="نام محصول"></asp:BoundField> <asp:BoundField DataField="Quantity" HeaderText="تعداد"></asp:BoundField> <asp:BoundField DataField="Price" HeaderText="قیمت"></asp:BoundField> </Columns> </asp:GridView> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">بستن</button> </div> </div> </div> </div> </form> </body>
حال به صفحه دستورات رفته و فضای نام موردنظر را به آن اضافه کنید و دستورات را در آن درج کنید.
using System; using System.Collections.Generic; using System.Data; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class GridviewPopup : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { BindGridView1(); } } protected void BindGridView1() { DataSet ds = new DataSet(); DataTable dt; DataRow dr; DataColumn pName; DataColumn pQty; DataColumn pPrice; int i = 0; dt = new DataTable(); pName = new DataColumn("Product_Name", Type.GetType("System.String")); pQty = new DataColumn("Quantity", Type.GetType("System.Int32")); pPrice = new DataColumn("Price", Type.GetType("System.Int32")); dt.Columns.Add(pName); dt.Columns.Add(pQty); dt.Columns.Add(pPrice); dr = dt.NewRow(); dr["Product_Name"] = "محصول شماره 1"; dr["Quantity"] = 2; dr["Price"] = 200; dt.Rows.Add(dr); dr = dt.NewRow(); dr["Product_Name"] = "محصول شماره 2"; dr["Quantity"] = 5; dr["Price"] = 480; dt.Rows.Add(dr); dr = dt.NewRow(); dr["Product_Name"] = "محصول شماره 3"; dr["Quantity"] = 8; dr["Price"] = 100; dt.Rows.Add(dr); dr = dt.NewRow(); dr["Product_Name"] = "محصول شماره 4"; dr["Quantity"] = 2; dr["Price"] = 500; dt.Rows.Add(dr); ds.Tables.Add(dt); GridView1.DataSource = ds.Tables[0]; GridView1.DataBind(); } protected void BindGrid2() { DataSet ds = new DataSet(); DataTable dt; DataRow dr; DataColumn pName; DataColumn pQty; DataColumn pPrice; int i = 0; dt = new DataTable(); pName = new DataColumn("Product_Name", Type.GetType("System.String")); pQty = new DataColumn("Quantity", Type.GetType("System.Int32")); pPrice = new DataColumn("Price", Type.GetType("System.Int32")); dt.Columns.Add(pName); dt.Columns.Add(pQty); dt.Columns.Add(pPrice); dr = dt.NewRow(); dr["Product_Name"] = "پیراهن مردانه"; dr["Quantity"] = 2; dr["Price"] = 200; dt.Rows.Add(dr); dr = dt.NewRow(); dr["Product_Name"] = "کفش ورزشی"; dr["Quantity"] = 5; dr["Price"] = 480; dt.Rows.Add(dr); dr = dt.NewRow(); dr["Product_Name"] = "تی شرت"; dr["Quantity"] = 8; dr["Price"] = 100; dt.Rows.Add(dr); dr = dt.NewRow(); dr["Product_Name"] = "شلوار جین"; dr["Quantity"] = 2; dr["Price"] = 500; dt.Rows.Add(dr); ds.Tables.Add(dt); GridView2.DataSource = ds.Tables[0]; GridView2.DataBind(); } protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e) { if (e.Row.RowType == DataControlRowType.DataRow) { string username = Convert.ToString(DataBinder.Eval(e.Row.DataItem, "Product_Name")); LinkButton lnkbtnresult = (LinkButton)e.Row.FindControl("lnkdelete"); BindGrid2(); } } }
خروجی:
خروجی برنامه بعد از نمایش Modal Pop-Up:
نکات قابل توجه:
1- در اینجا آموختید که چگونه می توانید در ASP.NET با استفاده از BootStrap یک modal pop-up نمایش دهید.
2- همینطور آموختید که چگونه میتوانید در کنار Grid، با استفاده از link-button یک Grid دیگر نمایش دهید.
- ASP.net
- 2k بازدید
- 5 تشکر