نحوه ایجاد Boot Strap Confirm Box در ASP.NET Web forms
پنجشنبه 18 تیر 1394در این مقاله خواهیم آموخت که چگونه می توانیم یک صفحه نمایشی Modal در GridView برای تائید پیغام خطا ایجاد کنیم.
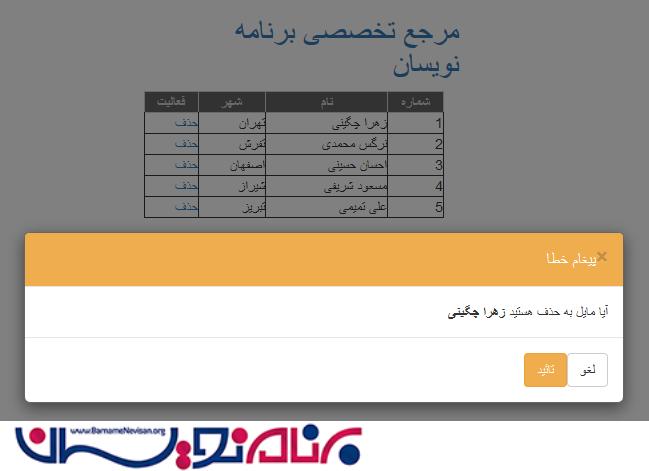
Bootstrap یک Framework محبوب HTML ،CSS و JavaScript برای ایجاد وب سایت های واکنش گرا است. در این مقاله نحوه استفاده از پیغام Bootstrap Confirm modal در ASP.NET را خواهیم آموخت. این که مسئله رایج است که وقتی ما بخواهیم هر رکوردی را حذف کنیم، قبل از حذف کامل یک پیغام جهت تائید حذف برای ما نمایش داده شود. در اینجا نشان می دهیم که چگونه می توانیم در زمانی که کاربر تمایل به حذف یک رکورد را داشت، یک صفحه Modal درون GridView Link برای او نمایش دهیم.
به منظور سادگی بیشتر، ما از یک کلاسی با تعدادی اطلاعات استفاده می کنیم. برای مجموعه اطلاعات GridView از پایگاه داده استفاده میکنیم.
using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace BootStrapConfirm { [Serializable()] public class Details { public int Id { get; set; } public string Name { get; set; } public string City { get; set; } public List<Details> GetDetails() { List<Details> Persons = new List<Details>(); Persons.Add(new Details { Id=1,Name= "زهرا چگینی", City="تهران" }); Persons.Add(new Details { Id = 2, Name = "نرگس محمدی", City = "تفرش" }); Persons.Add(new Details { Id = 3, Name = "احسان حسینی", City = "اصفهان" }); Persons.Add(new Details { Id = 4, Name = "مسعود شریفی", City = "شیراز" }); Persons.Add(new Details { Id = 5, Name = "علی تمیمی", City = "تبریز" }); return Persons; } } }
حال باید یک webform ConfirmDemo.aspx ایجاد کنیم.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="ConfirmDemo.aspx.cs" Inherits="BootStrapConfirm.ConfirmDemo" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>نمایش پیغام تائید</title> <script src="js/jquery-1.10.2.min.js"></script> <script src="js/bootstrap.min.js"></script> <script src="js/bootstrap-dialog.min.js"></script> <link href="css/bootstrap-dialog.min.css" rel="stylesheet" /> <link href="css/bootstrap.min.css" rel="stylesheet" /> <style> .row1 { padding: 4px 30px 4px 6px; border-bottom: 1px solid #b3b3b3; } .header { background: #646464; color: #d1d1d1; text-align: center; font-weight: bold; padding: 4px 30px 4px 6px; border-bottom: 1px solid #b3b3b3; } </style> </head> <body dir="rtl"> <form id="form1" runat="server"> <h2> <a href="http://www.barnamenevisan.org">مرجع تخصصی برنامه نویسان</a> </h2> <div class="container"> <asp:GridView ID="grdDemo" runat="server" AutoGenerateColumns="false" RowStyle-CssClass="row1" HeaderStyle-CssClass="header" OnRowCommand="grdDemo_RowCommand" OnRowDeleting="grdDemo_RowDeleting"> <Columns> <asp:TemplateField HeaderText="Id"> <ItemTemplate> <asp:Label ID="lblId" runat="server" Text='<%#Eval("Id") %>'></asp:Label> </ItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="Name"> <ItemTemplate> <asp:Label ID="lblName" runat="server" Text='<%#Eval("Name") %>'></asp:Label> </ItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="City"> <ItemTemplate> <asp:Label ID="lblCity" runat="server" Text='<%#Eval("City") %>'></asp:Label> </ItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="Action"> <ItemTemplate> <asp:LinkButton ID="lnkDelete" runat="server" CssClass="" OnClientClick='<%# string.Concat("if(!popup(this.id",",",Eval("ID"),",\"",Eval("Name"),"\"))return false; ") %>' Text="حذف" CommandArgument='<%# Eval("Id") %>' CommandName="Delete" ></asp:LinkButton> </ItemTemplate> </asp:TemplateField> </Columns> </asp:GridView> </div> <script> function popup(lnk, id, Name) { BootstrapDialog.confirm({ title: 'پیغام خطا', message: 'آیا مایل به حذف هستید <b>'+Name+'</b>', type: BootstrapDialog.TYPE_WARNING, closable: true, draggable: true, btnCancelLabel: 'لغو', btnOKLabel: 'تائید', btnOKClass: 'btn-warning', callback: function (result) { if (result) { javascript: __doPostBack('grdDemo$ctl02$lnkDelete', ''); } else { BootstrapDialog.closeAll(); } } }); } </script> </form> </body> </html>
در ادامه دستورات مربوطه را مشاهده می کنیم.
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace BootStrapConfirm { public partial class ConfirmDemo : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) gridbind(); } protected void grdDemo_RowCommand(object sender, GridViewCommandEventArgs e) { if (e.CommandName == "Delete") { LinkButton lnkView = (LinkButton)e.CommandSource; string dealId = lnkView.CommandArgument; List<Details> data = (List<Details>)ViewState["Data"]; data.RemoveAll(d => d.Id == Convert.ToInt32(dealId)); ViewState["Data"] = data; gridbind(); System.Web.UI.ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "AlertBox", "BootstrapDialog.alert('Record Deleted Successfully.');", true); //data. } } protected void gridbind() { if (ViewState["Data"] != null) { } else { Details d = new Details(); ViewState["Data"] = d.GetDetails(); } grdDemo.DataSource = (List<Details>)ViewState["Data"]; grdDemo.DataBind(); } protected void grdDemo_RowDeleting(object sender, GridViewDeleteEventArgs e) { } } }
در ادامه تصاویر حاصل از خروجی برنامه را مشاهده می کنید.
- ASP.net
- 2k بازدید
- 2 تشکر