ارسال پسورد تصادفی به ایمیل درASP.Net
سه شنبه 17 شهریور 1394در این مقاله آموزش می دهیم چگونه با استفاده از C# در ASP.Net پسورد تصادفی به ایمیل ارسال کنیم.
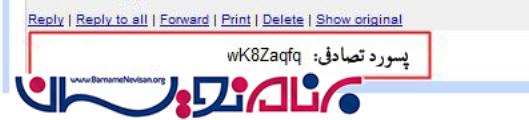
احتمالا در بسیاری از فرم های ثبت نام مشاهده نموده اید که تنها دو فیلد نام کاربری و ایمیل دارند و پسورد به ایمیل وارد شده ارسال می شود، در این مقاله می خواهیم چنین کاری انجام دهیم.
گام اول:
ویژوال استودیو را باز کرده و یک پروژه از نوع Empty Website با نام مناسب ایجاد می کنیم. در اینجا پروژه ای با نام randompass_demo ایجاد کردیم.
گام دوم:
در Solution Explorer روی پروژه راست کلیک کرده و با انتخاب Add و سپس New Item، یک Web form به نام Randompass_demo.aspx اضافه می کنیم. سپس به همین ترتیب، یک SQL Server Database در فولدر App_Data اضافه می کنیم.
طراحی دیتابیس:
گام سوم:
در Serveer explorer، روی نام دیتابیس ایجاد شده کلیک می کنیم و Tables و سپس Add New Table را انتخاب می کنیم و جدولی مانند جدول زیر با نام tbl_data ایجاد می کنیم.
گام چهارم:
فایل Randompass.aspx را باز کرده و طراحی برنامه کاربردی خود را به شکل زیر انجام می دهیم:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <style type="text/css"> .style1 { width: 196px; } .style2 { font-size: large; text-decoration: underline; text-align: left; color: #FF3300; } .auto-style1 { width: 196px; text-align: left; } .auto-style2 { width: 196px; text-align: left; height: 28px; } .auto-style3 { height: 28px; } </style> </head> <body> <form id="form1" runat="server"> <div> <table style="width:100%;" dir="rtl"> <tr> <td class="auto-style2"> <asp:Label ID="Label1" runat="server" ForeColor="Red" style="text-decoration: underline; font-weight: 700" Text="ارسال پسورد تصادفی به ایمیل"></asp:Label> </td> <td class="auto-style3"> </td> <td class="auto-style3"> </td> </tr> <tr> <td class="auto-style1"> نام کاربری:</td> <td> <asp:TextBox ID="TextBox1" runat="server" Width="207px"></asp:TextBox> </td> <td> </td> </tr> <tr> <td class="auto-style1"> آدرس ایمیل:</td> <td> <asp:TextBox ID="txtEmail" runat="server" Width="208px"></asp:TextBox> </td> <td> </td> </tr> <tr> <td class="auto-style1"> </td> <td> </td> <td> </td> </tr> <tr> <td class="auto-style1"> <asp:Label ID="lblMessage" runat="server"></asp:Label> </td> <td> <asp:Button ID="Button1" runat="server" onclick="Button1_Click1" Text="ثبت" /> </td> <td> </td> </tr> </table> </div> </form> </body> </html>
طراحی شما به شکل زیر خواهد بود:
گام پنجم:
سپس کد زیر را در randompass.aspx.cs می نویسیم:
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Net; using System.Net.Mail; using System.Data; using System.Data.SqlClient; public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { GeneratePassword(); } public string GeneratePassword() { string PasswordLength = "8"; string NewPassword = ""; string allowedChars = ""; allowedChars = "1,2,3,4,5,6,7,8,9,0"; allowedChars += "A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,V,W,X,Y,Z,"; allowedChars += "a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z,"; char[] sep = { ',' }; string[] arr = allowedChars.Split(sep); string IDString = ""; string temp = ""; Random rand = new Random(); for (int i = 0; i < Convert.ToInt32(PasswordLength); i++) { temp = arr[rand.Next(0, arr.Length)]; IDString += temp; NewPassword = IDString; } return NewPassword; } protected void Button1_Click1(object sender, EventArgs e) { // to save the username and password in database SqlConnection con = new SqlConnection(@"Data Source=.\SQLEXPRESS;AttachDbFilename=|DataDirectory|\Database.mdf;Integrated Security=True;User Instance=True"); SqlCommand cmd = new SqlCommand("insert into tbl_data(username,email) values (@username,@email)", con); cmd.Parameters.AddWithValue("username", TextBox1.Text); cmd.Parameters.AddWithValue("email", txtEmail.Text); con.Open(); int i = cmd.ExecuteNonQuery(); con.Close(); // to send the random password in email string strNewPassword = GeneratePassword().ToString(); MailMessage msg = new MailMessage(); msg.From = new MailAddress("info@barnamenevisan.org"); msg.To.Add(txtEmail.Text); msg.Subject = "پسورد تصادفی حساب کاربری شما"; msg.Body = "پسورد تصادفی:" + strNewPassword; msg.IsBodyHtml = true; SmtpClient smt = new SmtpClient(); smt.Host = "smtp.gmail.com"; System.Net.NetworkCredential ntwd = new NetworkCredential(); ntwd.UserName = "info@barnamenevisan.org"; //Your Email ID ntwd.Password = ""; // Your Password smt.UseDefaultCredentials = true; smt.Credentials = ntwd; smt.Port = 587; smt.EnableSsl = true; smt.Send(msg); lblMessage.Text = "ایمیل با موفقیت ارسال شد"; lblMessage.ForeColor = System.Drawing.Color.ForestGreen; } }
سپس متدی با نام ()GeneratePassword ایجاد کرده و آن را در این قسمت برای ارسال ایمیل فراخوانی می کنیم، زمانی که روی دکمه ثبت کلیک کنیم، کد بالا نام کاربری و ایمیل را ذخیره کرده و پسورد مربوطه را به ایمیل وارد شده ارسال می کند.
در اینجا، ما نام کاربری را "Barnamenevisan" و ایمیل "info@barnamenevisan.org" را وارد کرده ایم، که در دیتابیس ذخیره خواهد شد و پسورد تولید شده به ایمیل ارسال خواهد شد.
پسورد تولید شده:
داده های ذخیره شده در دیتابیس:
- ASP.net
- 2k بازدید
- 5 تشکر