آموزش فرم ثبت نام به صورت Ajax در MVC
پنجشنبه 21 آبان 1394در این مقاله قصد داریم یه توضیحی درباره ایجاد یک فرم ثبت نام به صورت کاملا Ajax ای و با استفاده از جاوا اسکریپت درتکنولوژی MVC بپردازیم
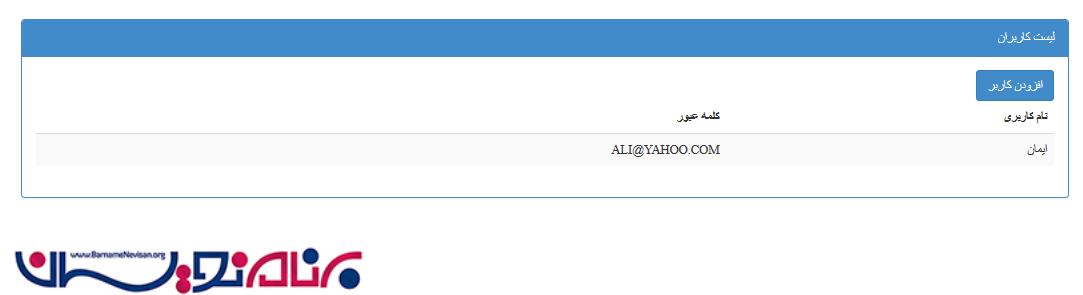
در ابتدا توضحی درباره این که Ajax چیست و چه کارایی دارد با هم صحبت میکنیم.
عبارت Ajax به طیف وسیعی از فن آوری های وب اشاره دارد که میتوانند برای پیاده سازی یک برنامه تحت وب بکار روند، فن آوریهایی که در پشت صحنه و در سرور فعالیت می کنند و در نتیجه با وضعیت جاری صفحه وب تداخلی ندارند
برای شروع یک پروژه از نوع MVC ایجاد میکنیم
وقتی یک فرم را به صورت POST به سرور میفرستیم، پاسخ آن با فرمت Json برمیگردد بنابراین ما باید یک کلاس برای ثبت داده ها هم به صورت POST و هم به صورت Json ایجاد کنیم که از کلاس زیر استفاده میکنیم
namespace AjaxForm.Serialization { public class ResponseData<T> { public T Data { get; set; } public string RedirectUrl { get; set; } public bool IsSuccess { get { return this.Data == null; } } } }
از آن جا که ما نیاز به بازگشت اطلاعات به صورت json داریم بایستی متد های ما خروجی از نوع JsonNetResult داشته باشند بنابر این بعد از نصب Newtonsoft.Json.5.0.8 با استفاده از Nuget به پیاده سازی کد زیر میپردازیم
using Newtonsoft.Json; using Newtonsoft.Json.Serialization; using System; using System.IO; using System.Web; using System.Web.Mvc; namespace AjaxForm.Serialization { public class JsonNetResult : JsonResult { public JsonNetResult() { Settings = new JsonSerializerSettings { ReferenceLoopHandling = ReferenceLoopHandling.Error, ContractResolver = new CamelCasePropertyNamesContractResolver() }; } public JsonSerializerSettings Settings { get; private set; } public override void ExecuteResult(ControllerContext context) { if (context == null) { throw new ArgumentNullException("context"); } if (this.JsonRequestBehavior == JsonRequestBehavior.DenyGet && string.Equals(context.HttpContext.Request.HttpMethod, "GET", StringComparison.OrdinalIgnoreCase)) { throw new InvalidOperationException("JSON GET is not allowed"); } HttpResponseBase response = context.HttpContext.Response; response.ContentType = string.IsNullOrEmpty(this.ContentType) ? "application/json" : this.ContentType; if (this.ContentEncoding != null) { response.ContentEncoding = this.ContentEncoding; } if (this.Data == null) { return; } var scriptSerializer = JsonSerializer.Create(this.Settings); using (var sw = new StringWriter()) { scriptSerializer.Serialize(sw, this.Data); response.Write(sw.ToString()); } } } }
حال ما میخواهیم اطلاعاتی به صورت Json برگردانده میشود را serialization کنیم برای یک کلاس به نام BaseController ایجاد کرده که از خود کلاس Controller ارث بری میکند
using AjaxForm.Serialization; using System.Linq; using System.Text; using System.Web.Mvc; namespace AjaxForm.Controllers { public class BaseController : Controller { public ActionResult NewtonSoftJsonResult(object data) { return new JsonNetResult { Data = data, JsonRequestBehavior = JsonRequestBehavior.AllowGet }; } public ActionResult CreateModelStateErrors() { StringBuilder errorSummary = new StringBuilder(); errorSummary.Append(@"<div class=""validation-summary-errors"" data-valmsg-summary=""true""><ul>"); var errors = ModelState.Values.SelectMany(x => x.Errors); foreach(var error in errors) { errorSummary.Append("<li>" + error.ErrorMessage + "</li>"); } errorSummary.Append("</ul></div>"); return Content(errorSummary.ToString()); } } }
حال ما نیاز به یک فایل جاوا اسکریپتی برای اعتبار سنجی فرم ها داریم که به صورت زیر پیاده سازی میکنیم
var Global = {}; Global.FormHelper = function (formElement, options, onSucccess, onError) { var settings = {}; settings = $.extend({}, settings, options); formElement.validate(settings.validateSettings); formElement.submit(function (e) { if (formElement.valid()) { $.ajax(formElement.attr("action"), { type: "POST", data: formElement.serializeArray(), success: function (result) { if (onSucccess === null || onSucccess === undefined) { if (result.isSuccess) { window.location.href = result.redirectUrl; } else { if (settings.updateTargetId) { $("#" + settings.updateTargetId).html(result.data); } } } else { onSucccess(result); } }, error: function (jqXHR, status, error) { if (onError !== null && onError !== undefined) { onError(jqXHR, status, error); $("#" + settings.updateTargetId).html(error); } }, complete: function () { } }); } e.preventDefault(); }); return formElement; };
حال برای ثبت اطلاعات ،یک کنتلرر به نام UserController که از کلاس BaseController ارث بری کرده است میسازیم که برای ثبت از فرمت Json استفاده کرده ایم.
using AjaxForm.Models; using AjaxForm.Serialization; using System.Collections.Generic; using System.Web.Mvc; namespace AjaxForm.Controllers { public class UserController : BaseController { public static List<UserViewModel> users = new List<UserViewModel>(); public ActionResult Index() { return View(users); } [HttpGet] public ActionResult AddUser() { UserViewModel model = new UserViewModel(); return View(model); } [HttpPost] public ActionResult AddUser(UserViewModel model) { if (ModelState.IsValid) { users.Add(model); return NewtonSoftJsonResult(new ResponseData<string> { RedirectUrl = @Url.Action("Index", "User") }); } return CreateModelStateErrors(); } }
حال روی متد Index راست کلیک کرده و یک View از آن ایجاد میکنیم
@model List<AjaxForm.Models.UserViewModel> <div class="panel panel-primary"> <div class="panel-heading panel-head">لیست کاربران </div> <div class="panel-body"> <div class="btn-group"> <a id="addUser" href="@Url.Action("AddUser")" class="btn btn-primary"> <i class="fa fa-plus"></i> Add User </a> </div> <table class="table table-striped"> <thead> <tr> <th>نام کاربری </th> <th>کلمه عبور </th> </tr> </thead> <tbody> @foreach (var item in Model) { <tr> <td>@item.UserName</td> <td>@item.Email</td> </tr> } </tbody> </table> </div> </div>
سپس همانند بالا روی متد AddUser راست کلیک کرده و در Add>View یک View ایجاد میکنیم
@model AjaxForm.Models.UserViewModel <div class="panel panel-primary"> <div class="panel-heading panel-head">افزودن کابر </div> <div id="frm-add-user" class="panel-body"> @using (Html.BeginForm()) { <div id="validation-summary"></div> <div class="form-horizontal"> <div class="form-group"> @Html.LabelFor(model => model.UserName, new { @class = "col-lg-2 control-label" }) <div class="col-lg-9"> @Html.TextBoxFor(model => model.UserName, new { @class = "form-control" }) @Html.ValidationMessageFor(model => model.UserName) </div> </div> <div class="form-group"> @Html.LabelFor(model => model.Email, new { @class = "col-lg-2 control-label" }) <div class="col-lg-9"> @Html.TextBoxFor(model => model.Email, new { @class = "form-control" }) @Html.ValidationMessageFor(model => model.Email) </div> </div> <div class="form-group"> <div class="col-lg-9"></div> <div class="col-lg-3"> <button class="btn btn-success" id="btnSubmit" type="submit"> Submit </button> </div> </div> </div> } </div> </div> @section scripts { @Scripts.Render("~/bundles/jqueryval","~/Scripts/global.js","~/Scripts/user_add_user.js") }
حال از برنامه اجرا میگریم
- ASP.net MVC
- 3k بازدید
- 8 تشکر