نحوه ایجاد انیمیشن در اندروید
شنبه 21 آذر 1394در این مقاله می خواهیم در مورد ساخت یک انیمیشن صحبت نماییم، در این مقاله یک مثال ساده از یک انیمیشن را برای شما قرار داده ایم.
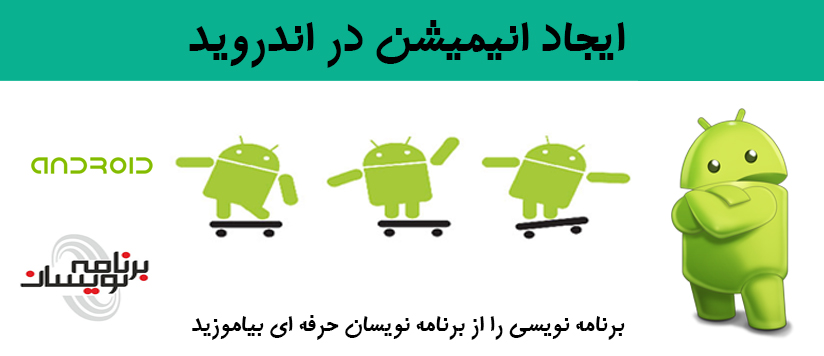
اگر دقت کرده باشید انیمیشن ها از قرار گرفتن چند عکس و یا نقاشی کنار هم در زمان های مختلف تصویر برداری شده است.
که اگر تمام این تصاویر کنار هم قرار بگیرند یک انیمیشن را برای ما تشکیل می دهد.
ابتدا یک پروژه ی جدید ایجاد نمایید داخل activity خود کد های زیر را قرار دهید.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#E6E6E6"> <ImageView android:layout_height="wrap_content" android:layout_width="wrap_content" android:id="@+id/imageAnimation" android:adjustViewBounds="true" /> <Button android:id="@+id/btnStart" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Start Animation" /> <Button android:id="@+id/btnStop" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Stop Animation" /> </LinearLayout>
در فولدر drawable عکس ها در موقعیت های مختلف عکس برداری شده است که در این پوشه قرار می گیرد.
ما از یک فایل xml برای قرار دادن عکس ها استفاده می نماییم که این فایل داخل پوشه ی anim قرار دارد.
<?xml version="1.0" encoding="utf-8"?> <animation-list xmlns:android="http://schemas.android.com/apk/res/android" android:oneshot="false"> <item android:drawable="@drawable/frame0" android:duration="50" /> <item android:drawable="@drawable/frame1" android:duration="50" /> <item android:drawable="@drawable/frame2" android:duration="50" /> <item android:drawable="@drawable/frame3" android:duration="50" /> <item android:drawable="@drawable/frame4" android:duration="50" /> <item android:drawable="@drawable/frame5" android:duration="50" /> <item android:drawable="@drawable/frame6" android:duration="50" /> <item android:drawable="@drawable/frame7" android:duration="50" /> <item android:drawable="@drawable/frame8" android:duration="50" /> <item android:drawable="@drawable/frame9" android:duration="50" /> <item android:drawable="@drawable/frame10" android:duration="50" /> <item android:drawable="@drawable/frame11" android:duration="50" /> <item android:drawable="@drawable/frame12" android:duration="50" /> <item android:drawable="@drawable/frame13" android:duration="50" /> <item android:drawable="@drawable/frame14" android:duration="50" /> <item android:drawable="@drawable/frame15" android:duration="50" /> <item android:drawable="@drawable/frame16" android:duration="50" /> <item android:drawable="@drawable/frame17" android:duration="50" /> <item android:drawable="@drawable/frame18" android:duration="50" /> <item android:drawable="@drawable/frame19" android:duration="50" /> <item android:drawable="@drawable/frame20" android:duration="50" /> <item android:drawable="@drawable/frame21" android:duration="50" /> <item android:drawable="@drawable/frame22" android:duration="50" /> <item android:drawable="@drawable/frame23" android:duration="50" /> <item android:drawable="@drawable/frame24" android:duration="50" /> <item android:drawable="@drawable/frame25" android:duration="50" /> <item android:drawable="@drawable/frame26" android:duration="50" /> <item android:drawable="@drawable/frame27" android:duration="50" /> <item android:drawable="@drawable/frame28" android:duration="50" /> <item android:drawable="@drawable/frame29" android:duration="50" /> <item android:drawable="@drawable/frame30" android:duration="50" /> <item android:drawable="@drawable/frame31" android:duration="50" /> <item android:drawable="@drawable/frame32" android:duration="50" /> <item android:drawable="@drawable/frame33" android:duration="50" /> <item android:drawable="@drawable/frame34" android:duration="50" /> <item android:drawable="@drawable/frame35" android:duration="50" /> </animation-list>
حالا داخل کلاس جاوا ابزار های زیر را تعریف نمایید:
ImageView animation; Button Start; Button Stop; Start=(Button)findViewById(R.id.btnStart); Stop=(Button)findViewById(R.id.btnStop); animation = (ImageView)findViewById(R.id.imageAnimation);
Imageview و Button را تعریف می نمایید.
حالا برای دکمه های تعریف شده یک رویداد تعریف می نمایید که دکمه ی شروع انیمیشن را اجرا نماید و دکمه ی توقف انیمیشن را متوقف خواهد کرد.
کل کد کلاس جاوا به صورت زیر خواهد بود:
import android.app.Activity; import android.graphics.drawable.AnimationDrawable; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.ImageView; public class Animation extends Activity { /** * Reference to the ImageView which will display the animation. */ ImageView animation; Button Start; Button Stop; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); Start = (Button) findViewById(R.id.btnStart); Stop = (Button) findViewById(R.id.btnStop); animation = (ImageView) findViewById(R.id.imageAnimation); animation.setBackgroundResource(R.drawable.animation); // the frame-by-frame animation defined as a xml file within the drawable folder final AnimationDrawable frameAnimation = (AnimationDrawable) animation.getBackground(); /* * NOTE: It's not possible to start the animation during the onCreate. * */ Start.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { // TODO Auto-generated method stub frameAnimation.start(); //Start the Animation } }); Stop.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { // TODO Auto-generated method stub frameAnimation.stop(); // Stop the Animation } }); } }
- Android
- 2k بازدید
- 6 تشکر