ایجاد PopUp Spinner با استفاده از فایل Json در اندروید
سه شنبه 29 دی 1394در این مقاله قصد داریم یک منوی آبشاری با استفاده از spinner ایجاد نماییم که اگر هر کدام را انتخاب کرد اطلاعات از یک فایل json خوانده شود و داخل یک Textview نمایش داده شود.
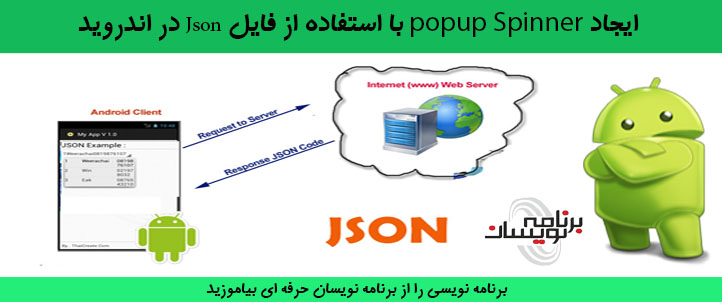
ابتدا یک پروژه ی جدید ایجاد نمایید و نام دلخواه برای خود پروژه و package مورد نظر بگذارید.
داخل کلاس MainActivity قطعه کد زیر را بنویسید:
package com.androidbegin.jsonspinnertutorial; import java.util.ArrayList; import org.json.JSONArray; import org.json.JSONObject; import android.os.AsyncTask; import android.os.Bundle; import android.app.Activity; import android.app.ProgressDialog; import android.util.Log; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.Spinner; import android.widget.TextView; public class MainActivity extends Activity { JSONObject jsonobject; JSONArray jsonarray; ProgressDialog mProgressDialog; ArrayList<String> worldlist; ArrayList<WorldPopulation> world; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); new DownloadJSON().execute(); } private class DownloadJSON extends AsyncTask<Void, Void, Void> { @Override protected Void doInBackground(Void... params) { world = new ArrayList<WorldPopulation>(); worldlist = new ArrayList<String>(); // JSON file URL address jsonobject = JSONfunctions .getJSONfromURL("http://www.androidbegin.com/tutorial/jsonparsetutorial.txt"); try { // Locate the NodeList name jsonarray = jsonobject.getJSONArray("worldpopulation"); for (int i = 0; i < jsonarray.length(); i++) { jsonobject = jsonarray.getJSONObject(i); WorldPopulation worldpop = new WorldPopulation(); worldpop.setRank(jsonobject.optString("rank")); worldpop.setCountry(jsonobject.optString("country")); worldpop.setPopulation(jsonobject.optString("population")); worldpop.setFlag(jsonobject.optString("flag")); world.add(worldpop); // Populate spinner with country names worldlist.add(jsonobject.optString("country")); } } catch (Exception e) { Log.e("Error", e.getMessage()); e.printStackTrace(); } return null; } @Override protected void onPostExecute(Void args) { // Locate the spinner in activity_main.xml Spinner mySpinner = (Spinner) findViewById(R.id.my_spinner); // Spinner adapter mySpinner .setAdapter(new ArrayAdapter<String>(MainActivity.this, android.R.layout.simple_spinner_dropdown_item, worldlist)); // Spinner on item click listener mySpinner .setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { @Override public void onItemSelected(AdapterView<?> arg0, View arg1, int position, long arg3) { // TODO Auto-generated method stub // Locate the textviews in activity_main.xml TextView txtrank = (TextView) findViewById(R.id.rank); TextView txtcountry = (TextView) findViewById(R.id.country); TextView txtpopulation = (TextView) findViewById(R.id.population); // Set the text followed by the position txtrank.setText("Rank : " + world.get(position).getRank()); txtcountry.setText("Country : " + world.get(position).getCountry()); txtpopulation.setText("Population : " + world.get(position).getPopulation()); } @Override public void onNothingSelected(AdapterView<?> arg0) { // TODO Auto-generated method stub } }); } } }
در این activity مورد نظر از یک فایل json در سرور استفاده می نماییم، که تابع json اطلاعات را در یک آرایه ی مورد نظر بر می گرداند، ما از یک AsyncTask برای بار گذاری اشیا json به یک آرایه ی رشته ای ایجاد کرده ایم، حال با انتخاب هر کدام از آیتم های مورد نظر باید اطلاعات از فایل json خوانده شود و داخل یک Textview نمایش داده شود.
فایل json به صورت زیر خواهد بود:
{ "worldpopulation": [ { "rank":1,"country":"China", "population":"1,354,040,000", "flag":"http://www.androidbegin.com/tutorial/flag/china.png" }, { "rank":2,"country":"India", "population":"1,210,193,422", "flag":"http://www.androidbegin.com/tutorial/flag/india.png" }, { "rank":3,"country":"United States", "population":"315,761,000", "flag":"http://www.androidbegin.com/tutorial/flag/unitedstates.png" }, { "rank":4,"country":"Indonesia", "population":"237,641,326", "flag":"http://www.androidbegin.com/tutorial/flag/indonesia.png" }, { "rank":5,"country":"Brazil", "population":"193,946,886", "flag":"http://www.androidbegin.com/tutorial/flag/brazil.png" }, { "rank":6,"country":"Pakistan", "population":"182,912,000", "flag":"http://www.androidbegin.com/tutorial/flag/pakistan.png" }, { "rank":7,"country":"Nigeria", "population":"170,901,000", "flag":"http://www.androidbegin.com/tutorial/flag/nigeria.png" }, { "rank":8,"country":"Bangladesh", "population":"152,518,015", "flag":"http://www.androidbegin.com/tutorial/flag/bangladesh.png" }, { "rank":9,"country":"Russia", "population":"143,369,806", "flag":"http://www.androidbegin.com/tutorial/flag/russia.png" }, { "rank":10,"country":"Japan", "population":"127,360,000", "flag":"http://www.androidbegin.com/tutorial/flag/japan.png" } ] }
یک کلاس دیگر ایجاد نمایید به صورت زیر:
package com.androidbegin.jsonspinnertutorial; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpPost; import org.apache.http.impl.client.DefaultHttpClient; import org.json.JSONException; import org.json.JSONObject; import android.util.Log; public class JSONfunctions { public static JSONObject getJSONfromURL(String url) { InputStream is = null; String result = ""; JSONObject jArray = null; // Download JSON data from URL try { HttpClient httpclient = new DefaultHttpClient(); HttpPost httppost = new HttpPost(url); HttpResponse response = httpclient.execute(httppost); HttpEntity entity = response.getEntity(); is = entity.getContent(); } catch (Exception e) { Log.e("log_tag", "Error in http connection " + e.toString()); } // Convert response to string try { BufferedReader reader = new BufferedReader(new InputStreamReader( is, "iso-8859-1"), 8); StringBuilder sb = new StringBuilder(); String line = null; while ((line = reader.readLine()) != null) { sb.append(line + "\n"); } is.close(); result = sb.toString(); } catch (Exception e) { Log.e("log_tag", "Error converting result " + e.toString()); } try { jArray = new JSONObject(result); } catch (JSONException e) { Log.e("log_tag", "Error parsing data " + e.toString()); } return jArray; } }
حالا یک activity به صورت زیر ایجاد نمایید و داخل آن یک spinner و textview قرار دهید:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" > <Spinner android:id="@+id/my_spinner" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/rank" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/my_spinner" /> <TextView android:id="@+id/country" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/rank" /> <TextView android:id="@+id/population" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/country" /> </RelativeLayout>
داخل androidmanifest مجوز دسترسی برای استفاده از اینترنت را وارد نمایید:
<uses-permission android:name="android.permission.INTERNET" />
خروجی به صورت زیر خواهد بود:
با استفاده از فایل json که در سرور قرار دارد شما مقدار های آن را به صورت شی برای spinner مورد نظر ارسال کردید.
- Android
- 2k بازدید
- 3 تشکر