ایجاد line number برای RichTextbox در #C
چهارشنبه 19 اسفند 1394در این مقاله به شما روشی آموزش داده میشود تا بتوانید line number برای یک RichTextBox ایجادکنید و مرحله به مرحله روش کار به شما توضیح داده خواهد شد.
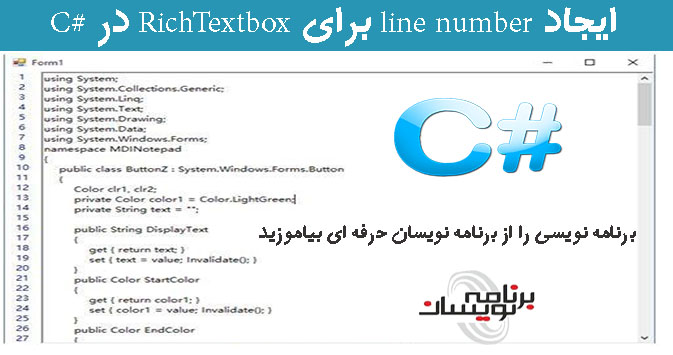
در کد بالا از تابع AddLineNumbers() استفاده شده است تا هر جایی از برنامه آن را فراخوانی کنیم.
از تابع getWidth() استفاده میکنیم تا عرضی مشخص، مطابق با تعداد خطوط برگرداند و عرض line numberها بطور خودکار افزایش یابد.
در این قسمت شما میتوانید کد مربوط به تابع AddLineNumbers() را مشاهده کنید.
public void AddLineNumbers() { // create & set Point pt to (0,0) Point pt = new Point(0, 0); // get First Index & First Line from richTextBox1 int First_Index = richTextBox1.GetCharIndexFromPosition(pt); int First_Line = richTextBox1.GetLineFromCharIndex(First_Index); // set X & Y coordinates of Point pt to ClientRectangle Width & Height respectively pt.X = ClientRectangle.Width; pt.Y = ClientRectangle.Height; // get Last Index & Last Line from richTextBox1 int Last_Index = richTextBox1.GetCharIndexFromPosition(pt); int Last_Line = richTextBox1.GetLineFromCharIndex(Last_Index); // set Center alignment to LineNumberTextBox LineNumberTextBox.SelectionAlignment = HorizontalAlignment.Center; // set LineNumberTextBox text to null & width to getWidth() function value LineNumberTextBox.Text = ""; LineNumberTextBox.Width = getWidth(); // now add each line number to LineNumberTextBox upto last line for (int i = First_Line; i <= Last_Line + 2; i++) { LineNumberTextBox.Text += i + 1 + "\n"; } }
مراحل:
مرحله اول: Visual Studio را باز میکنیم و یک Windows Forms Application جدید در C# ایجاد میکنیم.
مرحله دوم: یک RichTextBox به Form1 اضافه میکنیم و property آن را به صورت زیر تنظیم میکنیم:
شما میتوانید BackColor و ForeColorرا برای LineNumberTextBox به هر صورتی که میخواهید تنظیم کنید.
در اینجا میتوانید property Enable را false کنید،اما دیگر BackColor و ForeColorقابل مشاهده نخواهند بود.
BackColor و ForeColorو property های برای LineNumberTextBox را مانند بالا تنظیم میکنیم.
مرحله سوم: یک RichTextBox به Form1 اضافه میکنیم و خاصیت dock آن را در حالت Fill قرارمیدهیم.نام پیش فرض برای آن را richTextBox1 است.
مرحله چهارم: تابع AddLineNumbers() به کد Form 1 اضافه کنید.
مرحله پنچم: کد و eventهای زیر را به 1 Form اضافه کنید.
_Load:
1. private void Form1_Load(object sender, EventArgs e) 2. { 3. LineNumberTextBox.Font = richTextBox1.Font; 4. richTextBox1.Select(); 5. AddLineNumbers(); 6. }
_Resize:
1. private void Form1_Resize(object sender, EventArgs e) 2. { 3. AddLineNumbers(); 4. }
مرحله ششم: کد و eventهای زیر را به richTextBox1 اضافه کنید.
1. SelectionChanged
1. private void richTextBox1_SelectionChanged(object sender, EventArgs e) 2. { 3. Point pt = richTextBox1.GetPositionFromCharIndex(richTextBox1.SelectionStart); 4. if (pt.X == 1) 5. { 6. AddLineNumbers(); 7. } 8. }
2.VScroll:
1. private void richTextBox1_VScroll(object sender, EventArgs e) 2. { 3. LineNumberTextBox.Text = ""; 4. AddLineNumbers(); 5. LineNumberTextBox.Invalidate(); 6. }
5.FontChanged:
6. private void richTextBox1_FontChanged(object sender, EventArgs e) 7. { 8. LineNumberTextBox.Font = richTextBox1.Font; 9. richTextBox1.Select(); 10. AddLineNumbers(); 11. }
مرحله هفتم: eventها کد زیر را به LineNumberTextBox اضافه میکنیم.
_ MouseDown:
1. private void LineNumberTextBox_MouseDown(object sender, MouseEventArgs e) 2. { 3. richTextBox1.Select(); 4. LineNumberTextBox.DeselectAll(); 5. }
مرحله هشتم: Form خود را debug کنید و آن بررسی کنید.
کد کامل:
1. using System; 2. using System.Drawing; 3. using System.Windows.Forms; 4. 5. namespace LineNumbers_CSharp 6. { 7. public partial class Form1 : Form 8. { 9. public Form1() 10. { 11. InitializeComponent(); 12. } 13. 14. public int getWidth() 15. { 16. int w = 25; 17. // get total lines of richTextBox1 18. int line = richTextBox1.Lines.Length; 19. 20. if (line <= 99) 21. { 22. w = 20 + (int)richTextBox1.Font.Size; 23. } 24. else if (line <= 999) 25. { 26. w = 30 + (int)richTextBox1.Font.Size; 27. } 28. else 29. { 30. w = 50 + (int)richTextBox1.Font.Size; 31. } 32. 33. return w; 34. } 35. 36. public void AddLineNumbers() 37. { 38. // create & set Point pt to (0,0) 39. Point pt = new Point(0, 0); 40. // get First Index & First Line from richTextBox1 41. int First_Index = richTextBox1.GetCharIndexFromPosition(pt); 42. int First_Line = richTextBox1.GetLineFromCharIndex(First_Index); 43. // set X & Y coordinates of Point pt to ClientRectangle Width & Height respectively 44. pt.X = ClientRectangle.Width; 45. pt.Y = ClientRectangle.Height; 46. // get Last Index & Last Line from richTextBox1 47. int Last_Index = richTextBox1.GetCharIndexFromPosition(pt); 48. int Last_Line = richTextBox1.GetLineFromCharIndex(Last_Index); 49. // set Center alignment to LineNumberTextBox 50. LineNumberTextBox.SelectionAlignment = HorizontalAlignment.Center; 51. // set LineNumberTextBox text to null & width to getWidth() function value 52. LineNumberTextBox.Text = ""; 53. LineNumberTextBox.Width = getWidth(); 54. // now add each line number to LineNumberTextBox upto last line 55. for (int i = First_Line; i <= Last_Line + 2; i++) 56. { 57. LineNumberTextBox.Text += i + 1 + "\n"; 58. } 59. } 60. 61. private void Form1_Load(object sender, EventArgs e) 62. { 63. LineNumberTextBox.Font = richTextBox1.Font; 64. richTextBox1.Select(); 65. AddLineNumbers(); 66. } 67. 68. private void richTextBox1_SelectionChanged(object sender, EventArgs e) 69. { 70. Point pt = richTextBox1.GetPositionFromCharIndex(richTextBox1.SelectionStart); 71. if (pt.X == 1) 72. { 73. AddLineNumbers(); 74. } 75. } 76. 77. private void richTextBox1_VScroll(object sender, EventArgs e) 78. { 79. LineNumberTextBox.Text = ""; 80. AddLineNumbers(); 81. LineNumberTextBox.Invalidate(); 82. } 83. 84. private void richTextBox1_TextChanged(object sender, EventArgs e) 85. { 86. if (richTextBox1.Text == "") 87. { 88. AddLineNumbers(); 89. } 90. } 91. 92. private void richTextBox1_FontChanged(object sender, EventArgs e) 93. { 94. LineNumberTextBox.Font = richTextBox1.Font; 95. richTextBox1.Select(); 96. AddLineNumbers(); 97. } 98. 99. private void LineNumberTextBox_MouseDown(object sender, MouseEventArgs e) 100. { 101. richTextBox1.Select(); 102. LineNumberTextBox.DeselectAll(); 103. } 104. 105. private void Form1_Resize(object sender, EventArgs e) 106. { 107. AddLineNumbers(); 108. } 109. 110. } 111. }
- C#.net
- 2k بازدید
- 1 تشکر