عملیات CRUD با استفاده از AJX و Bootstrap در MVC
جمعه 15 مرداد 1395در این مقاله شما نحوه استفاده از CRUD در ASP.Net را با استفاده از AJAX و Bootstrap خواهید آموخت .
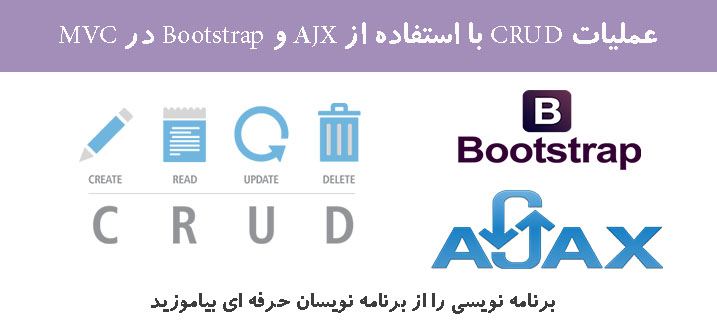
معرفی AJAX و Bootstrap :
AJAX (Asynchronous JavaScript and XML) در برنامه های تحت وب برای بروزرسانی قسمتی از صفحه موجود و بازیابی اطلاعات سرور ، بصورت جداگانه ، مورد استفاده قرار میگیرد .
AJAX کارایی برنامه های تحت وب را بالا برده و و برنامه ها را بیشتر تعاملی میکند .
Bootstrap یکی از محبوب ترین کتابخانه های HTML , CSS , JS برای توسعه دادن واکنش گرا
(Developing Responsive) می باشد .
یک پروژه ASP.Net webApplication بسازید .
حال ، روی پروژه کلیک راست کرده و به Manage Nuget بروید .
Bootstrap را جستجو کرده و نصب کنید .
بعد از نصب pakage ، خواهید دید که فولدر های Content و Scripts به solution Explorer اضافه میشود .
حال به سراغ پایگاه داده میرویم و یک جدول با نام Employee می سازیم .
بعد از ساختن جدول ، برای insert , Update , Select و Delete شروع به ساختن Stord Procedure میکنیم.
--Select Employees Create Procedure SelectEmployee as Begin Select * from Employee; End --Insert and Update Employee Create Procedure InsertUpdateEmployee ( @Id integer, @Name nvarchar(50), @Age integer, @State nvarchar(50), @Country nvarchar(50), @Action varchar(10) ) As Begin if @Action='Insert' Begin Insert into Employee(Name,Age,[State],Country) values(@Name,@Age,@State,@Country); End if @Action='Update' Begin Update Employee set Name=@Name,Age=@Age,[State]=@State,Country=@Country where EmployeeID=@Id; End End --Delete Employee Create Procedure DeleteEmployee ( @Id integer ) as Begin Delete Employee where EmployeeID=@Id; End
روی فولدر model راست کلیک کرده و کلاس Employee.cs را اضافه کنید .
Employee.cs Code
public class Employee { public int EmployeeID { get; set; } public string Name { get; set; } public int Age { get; set; } public string State { get; set; } public string Country { get; set; } }
حال ، در فولدر Model کلاسی دیگر با نام EmployeeDB.cs اضافه کنید . در این مثال ، ما از ADO.Net برای پردازش داده ها در پایگاه داده استفاده خواهیم کرد .
public class EmployeeDB { //declare connection string string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString; //Return list of all Employees public List<Employee> ListAll() { List<Employee> lst = new List<Employee>(); using(SqlConnection con=new SqlConnection(cs)) { con.Open(); SqlCommand com = new SqlCommand("SelectEmployee",con); com.CommandType = CommandType.StoredProcedure; SqlDataReader rdr = com.ExecuteReader(); while(rdr.Read()) { lst.Add(new Employee { EmployeeID=Convert.ToInt32(rdr["EmployeeId"]), Name=rdr["Name"].ToString(), Age = Convert.ToInt32(rdr["Age"]), State = rdr["State"].ToString(), Country = rdr["Country"].ToString(), }); } return lst; } } //Method for Adding an Employee public int Add(Employee emp) { int i; using(SqlConnection con=new SqlConnection(cs)) { con.Open(); SqlCommand com = new SqlCommand("InsertUpdateEmployee", con); com.CommandType = CommandType.StoredProcedure; com.Parameters.AddWithValue("@Id",emp.EmployeeID); com.Parameters.AddWithValue("@Name", emp.Name); com.Parameters.AddWithValue("@Age", emp.Age); com.Parameters.AddWithValue("@State", emp.State); com.Parameters.AddWithValue("@Country", emp.Country); com.Parameters.AddWithValue("@Action", "Insert"); i = com.ExecuteNonQuery(); } return i; } //Method for Updating Employee record public int Update(Employee emp) { int i; using (SqlConnection con = new SqlConnection(cs)) { con.Open(); SqlCommand com = new SqlCommand("InsertUpdateEmployee", con); com.CommandType = CommandType.StoredProcedure; com.Parameters.AddWithValue("@Id", emp.EmployeeID); com.Parameters.AddWithValue("@Name", emp.Name); com.Parameters.AddWithValue("@Age", emp.Age); com.Parameters.AddWithValue("@State", emp.State); com.Parameters.AddWithValue("@Country", emp.Country); com.Parameters.AddWithValue("@Action", "Update"); i = com.ExecuteNonQuery(); } return i; } //Method for Deleting an Employee public int Delete(int ID) { int i; using (SqlConnection con = new SqlConnection(cs)) { con.Open(); SqlCommand com = new SqlCommand("DeleteEmployee", con); com.CommandType = CommandType.StoredProcedure; com.Parameters.AddWithValue("@Id", ID); i = com.ExecuteNonQuery(); } return i; } }
یک Controller با نام HomeController به پروژه اضافه میکنیم .
HomeController را باز کرده و ActionMethode های زیر را به آن اضافه کنید .
public class HomeController : Controller { EmployeeDB empDB = new EmployeeDB(); // GET: Home public ActionResult Index() { return View(); } public JsonResult List() { return Json(empDB.ListAll(),JsonRequestBehavior.AllowGet); } public JsonResult Add(Employee emp) { return Json(empDB.Add(emp), JsonRequestBehavior.AllowGet); } public JsonResult GetbyID(int ID) { var Employee = empDB.ListAll().Find(x => x.EmployeeID.Equals(ID)); return Json(Employee, JsonRequestBehavior.AllowGet); } public JsonResult Update(Employee emp) { return Json(empDB.Update(emp), JsonRequestBehavior.AllowGet); } public JsonResult Delete(int ID) { return Json(empDB.Delete(ID), JsonRequestBehavior.AllowGet); } }
روی Index راست کلیک کرده و آن را به View اضافه کنید . حال به سراغ استفاده از Bootstrap وAJAX میرویم ، refrence های مورد نیاز را در view به head اضافه میکنیم . ما همچنین employee.js را اضافه میکنیم ، که شامل تمام کدهی AJAX میباشد ، و پیش نیازی است برای استفاده از کارکرد CRUD .
<script src="~/Scripts/jquery-1.9.1.js"></script> <script src="~/Scripts/bootstrap.js"></script> <link href="~/Content/bootstrap.css" rel="stylesheet" /> <script src="~/Scripts/employee.js"></script>
کدهای زیر را به view با نام Index.cshtml اضافه می کنیم .
<div class="container"> <h2>Employees Record</h2> <button type="button" class="btn btn-primary" data-toggle="modal" data-target="#myModal" onclick="clearTextBox();">Add New Employee</button><br /><br /> <table class="table table-bordered table-hover"> <thead> <tr> <th> ID </th> <th> Name </th> <th> Age </th> <th> State </th> <th> Country </th> <th> Action </th> </tr> </thead> <tbody class="tbody"> </tbody> </table> </div> <div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal">×</button> <h4 class="modal-title" id="myModalLabel">Add Employee</h4> </div> <div class="modal-body"> <form> <div class="form-group"> <label for="EmployeeId">ID</label> <input type="text" class="form-control" id="EmployeeID" placeholder="Id" disabled="disabled"/> </div> <div class="form-group"> <label for="Name">Name</label> <input type="text" class="form-control" id="Name" placeholder="Name"/> </div> <div class="form-group"> <label for="Age">Age</label> <input type="text" class="form-control" id="Age" placeholder="Age" /> </div> <div class="form-group"> <label for="State">State</label> <input type="text" class="form-control" id="State" placeholder="State"/> </div> <div class="form-group"> <label for="Country">Country</label> <input type="text" class="form-control" id="Country" placeholder="Country"/> </div> </form> </div> <div class="modal-footer"> <button type="button" class="btn btn-primary" id="btnAdd" onclick="return Add();">Add</button> <button type="button" class="btn btn-primary" id="btnUpdate" style="display:none;" onclick="Update();">Update</button> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> </div> </div> </div> </div>
در کد بالا ، ما یک دکمه برای اضافه کردن کارمندان جدید داریم . با کلیک بر روی آن ، یک modal که با استفاده از Bootstrap پیاده سازی شده است که شامل چندین فیلد از employee است ، باز می شود .
ما همچنین یک جدول اضافه میکنیم که با Ajxa پیاده سازی شده است .
Employee.js Code
//Load Data in Table when documents is ready $(document).ready(function () { loadData(); }); //Load Data function function loadData() { $.ajax({ url: "/Home/List", type: "GET", contentType: "application/json;charset=utf-8", dataType: "json", success: function (result) { var html = ''; $.each(result, function (key, item) { html += '<tr>'; html += '<td>' + item.EmployeeID + '</td>'; html += '<td>' + item.Name + '</td>'; html += '<td>' + item.Age + '</td>'; html += '<td>' + item.State + '</td>'; html += '<td>' + item.Country + '</td>'; html += '<td><a href="#" onclick="return getbyID(' + item.EmployeeID + ')">Edit</a> | <a href="#" onclick="Delele(' + item.EmployeeID + ')">Delete</a></td>'; html += '</tr>'; }); $('.tbody').html(html); }, error: function (errormessage) { alert(errormessage.responseText); } }); } //Add Data Function function Add() { var res = validate(); if (res == false) { return false; } var empObj = { EmployeeID: $('#EmployeeID').val(), Name: $('#Name').val(), Age: $('#Age').val(), State: $('#State').val(), Country: $('#Country').val() }; $.ajax({ url: "/Home/Add", data: JSON.stringify(empObj), type: "POST", contentType: "application/json;charset=utf-8", dataType: "json", success: function (result) { loadData(); $('#myModal').modal('hide'); }, error: function (errormessage) { alert(errormessage.responseText); } }); } //Function for getting the Data Based upon Employee ID function getbyID(EmpID) { $('#Name').css('border-color', 'lightgrey'); $('#Age').css('border-color', 'lightgrey'); $('#State').css('border-color', 'lightgrey'); $('#Country').css('border-color', 'lightgrey'); $.ajax({ url: "/Home/getbyID/" + EmpID, typr: "GET", contentType: "application/json;charset=UTF-8", dataType: "json", success: function (result) { $('#EmployeeID').val(result.EmployeeID); $('#Name').val(result.Name); $('#Age').val(result.Age); $('#State').val(result.State); $('#Country').val(result.Country); $('#myModal').modal('show'); $('#btnUpdate').show(); $('#btnAdd').hide(); }, error: function (errormessage) { alert(errormessage.responseText); } }); return false; } //function for updating employee's record function Update() { var res = validate(); if (res == false) { return false; } var empObj = { EmployeeID: $('#EmployeeID').val(), Name: $('#Name').val(), Age: $('#Age').val(), State: $('#State').val(), Country: $('#Country').val(), }; $.ajax({ url: "/Home/Update", data: JSON.stringify(empObj), type: "POST", contentType: "application/json;charset=utf-8", dataType: "json", success: function (result) { loadData(); $('#myModal').modal('hide'); $('#EmployeeID').val(""); $('#Name').val(""); $('#Age').val(""); $('#State').val(""); $('#Country').val(""); }, error: function (errormessage) { alert(errormessage.responseText); } }); } //function for deleting employee's record function Delele(ID) { var ans = confirm("Are you sure you want to delete this Record?"); if (ans) { $.ajax({ url: "/Home/Delete/" + ID, type: "POST", contentType: "application/json;charset=UTF-8", dataType: "json", success: function (result) { loadData(); }, error: function (errormessage) { alert(errormessage.responseText); } }); } } //Function for clearing the textboxes function clearTextBox() { $('#EmployeeID').val(""); $('#Name').val(""); $('#Age').val(""); $('#State').val(""); $('#Country').val(""); $('#btnUpdate').hide(); $('#btnAdd').show(); $('#Name').css('border-color', 'lightgrey'); $('#Age').css('border-color', 'lightgrey'); $('#State').css('border-color', 'lightgrey'); $('#Country').css('border-color', 'lightgrey'); } //Valdidation using jquery function validate() { var isValid = true; if ($('#Name').val().trim() == "") { $('#Name').css('border-color', 'Red'); isValid = false; } else { $('#Name').css('border-color', 'lightgrey'); } if ($('#Age').val().trim() == "") { $('#Age').css('border-color', 'Red'); isValid = false; } else { $('#Age').css('border-color', 'lightgrey'); } if ($('#State').val().trim() == "") { $('#State').css('border-color', 'Red'); isValid = false; } else { $('#State').css('border-color', 'lightgrey'); } if ($('#Country').val().trim() == "") { $('#Country').css('border-color', 'Red'); isValid = false; } else { $('#Country').css('border-color', 'lightgrey'); } return isValid; }
در خروجی خواهیم دید :
آموزش asp.net mvc
- ASP.net MVC
- 2k بازدید
- 4 تشکر