صفحه بندی (Pagination) با استفاده از JavaScript در MVC
شنبه 8 آبان 1395در این مقاله ، ما نحوه ساخت صفحه بندی (Pagination) را با استفاده از JavaScript در mvc خواهیم آموخت.
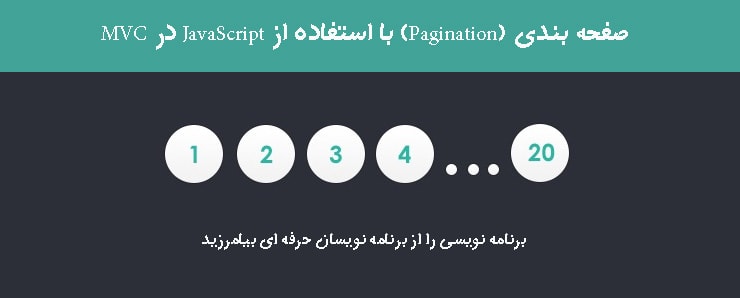
در این مقاله ، چگونگی Pagination با بازیابی اطلاعات در MVC با استفاده از jQuery را خواهیم دید :
1. Visual Studio را باز کرده و New Project را بزنید .
2. در قسمت #ASP.Net Web Application ، Visual C را انتخاب کنید .
3. حال در پنجره باز شده ، یک پروژه Empty را انتخاب کرده و تیک MVC را بزنید .
4. حال ، روی Solution خود راست کلیک کرده و یک پروژه دیگر تحت عنوان Class library اضافه کنید . (ما از Entity Framework استفاده خواهیم کرد . )
5. یک کلاس اضافه کنید و کد های زیر را در آن قرار دهید .
public class Users { [Key] public int Id { get; set; } public string Name { get; set; } public string Address { get; set; } public string Phone_Number { get; set; } }
حال ، روی Class library خود راست کلیک کرده و با استفاده از Entity Framework ، NuGet را نصب کنید .
حال ، یک فولدر در class library ایجاد کرده و نام آن را Context بگذارید و یک کلاس همانند زیر به آن اضافه کنید:
public class DBContext : DbContext { public DbSet<Users> ObjUser { get; set; } }
در Web.Config پروژه ، Connection String زیر را قرار دهید :
توجه داشته باشید که قسمت هایی از کد زیر باید توسط خود شما تکمیل شود .
<connectionStrings> <add name="DBContext" connectionString="Your Connection string" providerName="System.Data.SqlClient" /> </connectionStrings>
حال ، در refrence پروژه ، Class library را اضافه کنید و پروژه را build کنید .
حال ، روی پروژه کلیک راست کرده و Entity Framework را با استفاده از NuGet نصب کنید .
یک Empty Controller را به فولدر Controller اضافه کنید .
متد ActionResult زیر را به Controller خود اضافه کنید .
public ActionResult Index() { using (DBContext context = new DBContext()) { var Users = context.ObjUser.Take(5).ToList(); //At first i will show only 5 data per page so i had used Take(5) int UsersCount= Convert.ToInt32(Math.Ceiling((double)context.ObjUser.Count() / 5)); //Now calulating the required buttons for pagination TempData["Users"] = Users; //Passing User details to view with TempData ViewBag.UsersCount = UsersCount; //Count of button to be displayed } return View(); }
حال در View متد Index ، اطلاعات بازیابی شده کاربر را در یک جدول نمایش خواهیم داد .
@{ ViewBag.Title = "Index"; var UsersData = TempData["Users"]; //Assigning the list of users to a variable } <script src="https://code.jquery.com/jquery-3.1.0.min.js" integrity="sha256-cCueBR6CsyA4/9szpPfrX3s49M9vUU5BgtiJj06wt/s=" crossorigin="anonymous"></script> <h2>Index</h2> <div> <table class="tablesorter"> <thead> <tr> <th>Name</th> <th>Address</th> <th>Phone_Number</th> </tr> </thead> <tbody id="tbldisplay"> @foreach (var p in (List<PaginationEntity.Users>)UsersData) //PaginationEntity is my Class Library Name and Users is my model in that Libraray { <tr> <td>@p.Name</td> <td>@p.Address</td> <td>@p.Phone_Number</td> </tr> } </tbody> </table> <form> //Here the number of buttons is to be displayed according the no os users shown per page <div id="SHowButton"> @for (int i = 1; i <= ViewBag.UsersCount; i++) { //SortData is a javascript function which is used for retrieving next or previous data according to page number <input type="button" id="@i" value="@i" onclick="SortData(@i)" /> } </div> //Display is another Javascript Function used for showing number of users according to the value selected from dropdown <select class="pagesize" onchange="Display()" id="drpdwn"> <option selected="selected" value="5">5</option> <option value="10">10</option> <option value="20">20</option> <option value="30">30</option> <option value="40">40</option> </select> <input type="text" id="Search"/> //Used for searching user on keypress </form> </div>
حال ، Display Function را اضافه خواهیم کرد ، که لیست کاربران را فراهم میآورد ، که Count مقداری خواهد بود که از dropdown انتخاب میشود .
<script> function Display() { //Passing the value of dropdown to method Page in my Controller var data = $("#drpdwn").val(); //Here Home is my Controller and Page is JsonResult Method $.get("/Home/Page", { NumberOfData: data }, function (response) { //NumberOfData is just an object whose value will be - value of dropdown //In response i will get the values returned by Page Method in controller //I had returned users and Button Count if (response != null) { $("#tbldisplay").empty(); $("#SHowButton").empty(); var datacontent = "" //appending the returned value for (var i = 0; i < response.user.length; i++) { //response.user is one of the returned value from method datacontent += "<tr><td>" + response.user[i].Name + "</td><td>" + response.user[i].Address + "</td><td>" + response.user[i].Phone_Number + "</td></tr>"; } var ButtonContent = ""; alert(response.CountUser); if (response.CountUser != 1) { for (var d = 1; d <= response.CountUser; d++) { // response.CountUser is another returned value from method ButtonContent += "<input type='button' id=" + d + " value=" + d + " onclick='SortData(" + d + ")' />"; } $("#SHowButton").append(ButtonContent); } $("#tbldisplay").append(datacontent); } }); } </script>
حال ، متد JsonResult با نام Page را به پروژه اضافه کنید .
//as i am retreving data so httpget [HttpGet] public JsonResult Page(int NumberOfData) //the object name should be same as used in JQuerry above it will get the value of dropdown { using (DBContext context = new DBContext()) { var Users = context.ObjUser.Take(NumberOfData).ToList(); //retreiving that much count of data int UsersCount = Convert.ToInt32(Math.Ceiling((double)context.ObjUser.Count() / NumberOfData)); //again for required button count var Result = new { user=Users,CountUser=UsersCount}; //here i am returning multiple data to my jquerry user and CountUser return Json(Result, JsonRequestBehavior.AllowGet); } }
حال ، برای گرفتن داده های قبلی و بعدی با کلیک بر روی دکمه ، همانند زیر Javascriptی را به پروژه اضافه میکنیم :
function SortData(number) //number will get the clicked button's number { var data = $("#drpdwn").val(); //passing current value of dropdwon //SortData is my method on home controller $.get("/Home/SortData",{DrpDwnValue:data,PageNumber:number},function(response){ if (response != null) { //appending the fetched value to the table $("#tbldisplay").empty(); var datacontent = "" for (var i = 0; i < response.length; i++) { datacontent += "<tr><td>" + response[i].Name + "</td><td>" + response[i].Address + "</td><td>" + response[i].Phone_Number + "</td></tr>"; } $("#tbldisplay").append(datacontent); } }); }
حال ، یک متد برای گرفتن لیست کاربران به پروژه اضافه میکنیم ، با کلیک بر روی دکمه :
[HttpGet] public JsonResult SortData(int DrpDwnValue,int PageNumber) //match the name and order of the parameters with your javacript above { using (DBContext context = new DBContext()) { //here for skipping the current data displayed you have to use the OrderBy function of the IQueryable //This will skip the current data and move to next or previous data according to the button you have clicked var Users = context.ObjUser.OrderBy(x => x.Id).Skip(DrpDwnValue * (PageNumber - 1)).Take(DrpDwnValue).ToList(); return Json(Users, JsonRequestBehavior.AllowGet); } }
برای جستجو :
از تابع KeyUp جاوا اسکریپت ، برای جستجوی متن جاری و تطابق با هر چیزی که در فیلد پر شده است از کد زیر استفاده میکنیم :
$("#Search").keyup(function (e) { clearTimeout($.data(this, 'timer')); if (e.keyCode == 13) MatchData(true); else $(this).data('timer', setTimeout(MatchData, 50)); //sets the timer between the key press from keyboard and the search }) function MatchData(force) { var event = $("#Search").val(); //fetching the value from the textbox $.get("/Home/SearchData", function (response) { //console.log(response); if (response.length) { $("#tbldisplay").empty(); var datacontent = ""; for (var i = 0; i < response.length; i++) { //Only the matching value will be displayed if ((response[i].Name).indexOf(event) != -1 || (response[i].Address).indexOf(event) != -1 || (response[i].Phone_Number).indexOf(event) != -1) //Name , Address and Phone_number are my attributes in User Table { datacontent += "<tr><td>" + response[i].Name + "</td><td>" + response[i].Address + "</td><td>" + response[i].Phone_Number + "</td></tr>"; } } $("#tbldisplay").append(datacontent); }); }
Action Method :
[HttpGet] public JsonResult SearchData() { using (DBContext context = new DBContext()) { var Users = context.ObjUser.ToList(); return Json(Users, JsonRequestBehavior.AllowGet); } }
داده هایی را وارد کردیم ، در خروجی خواهیم داشت :
آموزش asp.net mvc
- ASP.net MVC
- 3k بازدید
- 6 تشکر