شروع کار با MongoDB در ASP.NET Core
یکشنبه 27 خرداد 1397در این مقاله می خواهیم درباره ی روند ساخت یک اپلیکیشن ساده در ASP.NET Core که با پایگاه داده ی MongoDB در ارتباط است بحث کنیم. این اپلیکیشن عملیات CRUD در یک پایگاه داده ی Mongo را انجام می دهد و سپس جزئیات را در یک جدول نمایش می دهد.
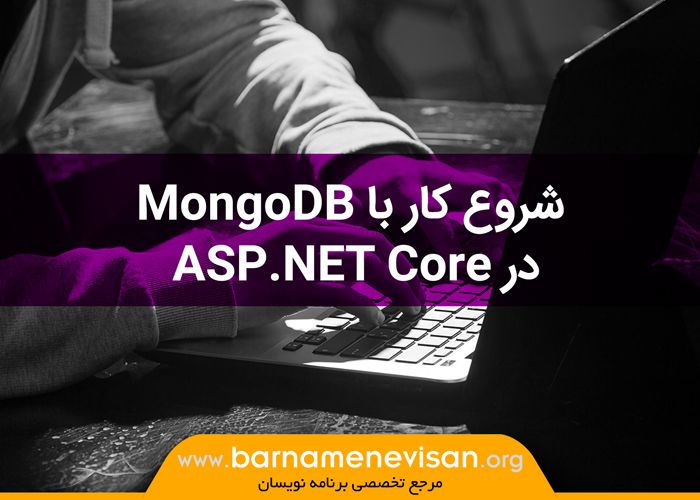
معرفی
در این مقاله می خواهیم درباره ی روند ساخت یک اپلیکیشن ساده در ASP.NET Core که با پایگاه داده ی MongoDB در ارتباط است بحث کنیم.
شرح
این اپلیکیشن عملیات CRUD در یک پایگاه داده ی Mongo را انجام می دهد و سپس جزئیات را در یک جدول نمایش می دهد.
ابزار های Third-party استفاده شده
Robo 3T – یک ابزار third-party است که یک ابزار مدیریتی MongoDB سبک را ارائه می دهد.
پیاده سازی تنظیمات اولیه ی MongoDB
اگر شما MongoDB exe را نصب نکرده اید آن را از MongoDB Download Center دانلود کنید.
پس از نصب پایگاه داده برای دسترسی به MongoDB باید پروسه ی MongoDB را شروع کنیم.
برای شروع MongoDB در command prompt ، mongod.exe را اجرا کنید. اطمینان حاصل کنید که command prompt را از پوشه ی installation در MongoDB اجرا می کنید.
به صورت پیش فرض مسیر نصب روی C:\Program Files\MongoDB\Server\3.6\bin\ تنظیم شده است علاوه بر این ما یک دایرکتوری داده برای ذخیره سازی همه ی داده ها در MongoDB نیاز داریم. کاربر می تواند مسیر فایل های داده را با استفاده از گزینه ی dbpath- به mongod.exe تنظیم کند.
شما می توانید استفاده از Robo 3T را پس از شروع پروسه ی MongoDB آغاز کنید.
ما برای این دمو یک collection به نام Customers با سه ستون ساخته ایم.
از آنجایی که پایگاه داده آماده است ساخت اپلیکیشن را شروع می کنیم. مقاله را برای ساخت یک اپلیکیشن نمونه دنبال کنید.
در ادامه یک نمای نمونه اپلیکیشنی که می خواهیم بسازیم وجود دارد.
یک پروژه ی جدید در Visual Studio بسازید.
template را ASP.NET Core MVC Web Application انتخاب کنید.
برای تعامل با MongoDB از طریق کد C# نیاز داریم که NET MongoDB Driver. ، که یک تعامل ناهمگام با MongoDB را ارائه می دهد، نصب کنیم. دستورات NuGet زیر را برای اضافه کردن driver به پروژه استفاده کرده ایم.
Install-Package Microsoft.EntityFrameworkCore.Tools -Version 2.0.1 Install-Package MongoDB.Driver -Version 2.5.0
کلاس Model
اجازه دهید یک کلاس موجودیت به نام “Customer” بسازیم که با شمای جدول Customers در پایگاه داده متناسب باشد.
public class Customer { [BsonId] public ObjectId Id { get; set; } [BsonElement] public int CustomerId { get; set; } [BsonElement] public string CustomerName { get; set; } [BsonElement] public string Address { get; set; } }
کلاس شامل خصوصیت Id از نوع ObjectId می باشد این خصوصیت برای تطبیق یک آیتم در collection های MongoDB استفاده می شود. ما همچنین یک ویژگی دیگر به نام BsonElement داریم که برای نشان دادن یک عنصر در collection های MongoDB به کار گرفته می شود.
متد های Controller
در Controller ما کدهایی برای خواندن ، ویرایش، ساخت و حذف رکوردها از MongoDB اضافه خواهیم کرد.کدها را برای بازیابی جزئیات پایگاه داده به یک متد معمول منتقل کرده ایم.
public class HomeController : Controller { private IMongoDatabase mongoDatabase; //Generic method to get the mongodb database details public IMongoDatabase GetMongoDatabase() { var mongoClient = new MongoClient("mongodb://localhost:27017"); return mongoClient.GetDatabase("CustomerDB"); } [HttpGet] public IActionResult Index() { //Get the database connection mongoDatabase = GetMongoDatabase(); //fetch the details from CustomerDB and pass into view var result = mongoDatabase.GetCollection<Customer>("Customers").Find(FilterDefinition<Customer>.Empty).ToList(); return View(result); } [HttpGet] public IActionResult Create() { return View(); } [HttpPost] public IActionResult Create(Customer customer) { try { //Get the database connection mongoDatabase = GetMongoDatabase(); mongoDatabase.GetCollection<Customer>("Customers").InsertOne(customer); } catch (Exception ex) { throw; } return RedirectToAction("Index"); } [HttpGet] public IActionResult Details(int? id) { if (id == null) { return NotFound(); } //Get the database connection mongoDatabase = GetMongoDatabase(); //fetch the details from CustomerDB and pass into view Customer customer = mongoDatabase.GetCollection<Customer>("Customers").Find<Customer>(k => k.CustomerId == id).FirstOrDefault(); if (customer == null) { return NotFound(); } return View(customer); } [HttpGet] public IActionResult Delete(int? id) { if (id == null) { return NotFound(); } //Get the database connection mongoDatabase = GetMongoDatabase(); //fetch the details from CustomerDB and pass into view Customer customer = mongoDatabase.GetCollection<Customer>("Customers").Find<Customer>(k => k.CustomerId == id).FirstOrDefault(); if (customer == null) { return NotFound(); } return View(customer); } [HttpPost] public IActionResult Delete(Customer customer) { try { //Get the database connection mongoDatabase = GetMongoDatabase(); //Delete the customer record var result = mongoDatabase.GetCollection<Customer>("Customers").DeleteOne<Customer>(k => k.CustomerId == customer.CustomerId); if (result.IsAcknowledged == false) { return BadRequest("Unable to Delete Customer " + customer.CustomerId); } } catch (Exception ex) { throw; } return RedirectToAction("Index"); } [HttpGet] public IActionResult Edit(int? id) { if (id == null) { return NotFound(); } //Get the database connection mongoDatabase = GetMongoDatabase(); //fetch the details from CustomerDB based on id and pass into view var customer = mongoDatabase.GetCollection<Customer>("Customers").Find<Customer>(k => k.CustomerId == id).FirstOrDefault(); if (customer == null) { return NotFound(); } return View(customer); } [HttpPost] public IActionResult Edit(Customer customer) { try { //Get the database connection mongoDatabase = GetMongoDatabase(); //Build the where condition var filter = Builders<Customer>.Filter.Eq("CustomerId", customer.CustomerId); //Build the update statement var updatestatement = Builders<Customer>.Update.Set("CustomerId", customer.CustomerId); updatestatement = updatestatement.Set("CustomerName", customer.CustomerName); updatestatement = updatestatement.Set("Address", customer.Address); //fetch the details from CustomerDB based on id and pass into view var result = mongoDatabase.GetCollection<Customer>("Customers").UpdateOne(filter, updatestatement); if (result.IsAcknowledged == false) { return BadRequest("Unable to update Customer " + customer.CustomerName); } } catch (Exception ex) { throw; } return RedirectToAction("Index"); } public IActionResult About() { ViewData["Message"] = "Your application description page."; return View(); } public IActionResult Contact() { ViewData["Message"] = "Your contact page."; return View(); } public IActionResult Error() { return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier }); } }
کد برای View های MVC
از آنجایی که این مقاله چیزی بیش از یک دمو از MongoDB است ما از گزینه ی scaffolding که در MVC برای ساخت View دردسترس است استفاده کرده ایم. شما می توانید این مورد را براساس نیاز های خود تغییر دهید.
InDEX VIEW
@model IEnumerable<AspNetCoreMVCMongoDBDemo.Models.Customer> @{ ViewData["Title"] = "Index"; } <h2>Index</h2> <p> <a asp-action="Create">Create New</a> </p> <table class="table table-bordered" style="width:600px"> <thead> <tr> <th> @Html.DisplayNameFor(model => model.CustomerId) </th> <th> @Html.DisplayNameFor(model => model.CustomerName) </th> <th> @Html.DisplayNameFor(model => model.Address) </th> <th>Actions</th> </tr> </thead> <tbody> @foreach (var item in Model) { <tr> <td> @Html.DisplayFor(modelItem => item.CustomerId) </td> <td> @Html.DisplayFor(modelItem => item.CustomerName) </td> <td> @Html.DisplayFor(modelItem => item.Address) </td> <td> @Html.ActionLink("Edit", "Edit", new { id = item.CustomerId }) | @Html.ActionLink("Details", "Details", new { id = item.CustomerId }) | @Html.ActionLink("Delete", "Delete", new { id = item.CustomerId }) </td> </tr> } </tbody> </table>
VIEW ساخت
@model AspNetCoreMVCMongoDBDemo.Models.Customer @{ ViewData["Title"] = "Create"; } <h2>Create Customer Details</h2> <hr /> <div class="row"> <div class="col-md-4"> <form asp-action="Create"> <div asp-validation-summary="ModelOnly" class="text-danger"></div> <div class="form-group"> <label asp-for="CustomerId" class="control-label"></label> <input asp-for="CustomerId" class="form-control" /> <span asp-validation-for="CustomerId" class="text-danger"></span> </div> <div class="form-group"> <label asp-for="CustomerName" class="control-label"></label> <input asp-for="CustomerName" class="form-control" /> <span asp-validation-for="CustomerName" class="text-danger"></span> </div> <div class="form-group"> <label asp-for="Address" class="control-label"></label> <input asp-for="Address" class="form-control" /> <span asp-validation-for="Address" class="text-danger"></span> </div> <div class="form-group"> <input type="submit" value="Create" class="btn btn-default" /> </div> </form> </div> </div> <div> <a asp-action="Index">Back to List</a> </div> @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} }
VIEW حذف
@model AspNetCoreMVCMongoDBDemo.Models.Customer @{ Layout = "_Layout"; } <h4>Delete Customer</h4> <div class="row"> <div class="col-md-4"> <form asp-action="Delete"> <label class="control-label">Are you sure to delete </label> <input asp-for="CustomerId" class="form-control" readonly /> <div class="form-group"> <input type="submit" value="Delete" class="btn btn-default" /> </div> </form> </div> </div> <div> <a asp-action="Index">Back to List</a> </div>
VIEW جزئیات
@model AspNetCoreMVCMongoDBDemo.Models.Customer @{ ViewData["Title"] = "Details"; } <div> <h4>Customer Details</h4> <hr /> <dl class="dl-horizontal"> <dt> @Html.DisplayNameFor(model => model.CustomerId) </dt> <dd> @Html.DisplayFor(model => model.CustomerId) </dd> <dt> @Html.DisplayNameFor(model => model.CustomerName) </dt> <dd> @Html.DisplayFor(model => model.CustomerName) </dd> <dt> @Html.DisplayNameFor(model => model.Address) </dt> <dd> @Html.DisplayFor(model => model.Address) </dd> </dl> </div> <div> <a asp-action="Index">Back to List</a> </div>
VIEW ویرایش
@model AspNetCoreMVCMongoDBDemo.Models.Customer @{ Layout = "_Layout"; } @{ ViewData["Title"] = "Details"; } <h2>Edit Customer Details</h2> <hr /> <div class="row"> <div class="col-md-4"> <form asp-action="Edit"> <div asp-validation-summary="ModelOnly" class="text-danger"></div> <div class="form-group"> <label asp-for="CustomerId" class="control-label"></label> <input asp-for="CustomerId" class="form-control" /> </div> <div class="form-group"> <label asp-for="CustomerName" class="control-label"></label> <input asp-for="CustomerName" class="form-control" /> <span asp-validation-for="CustomerName" class="text-danger"></span> </div> <div class="form-group"> <label asp-for="Address" class="control-label"></label> <input asp-for="Address" class="form-control" /> <span asp-validation-for="Address" class="text-danger"></span> </div> <div class="form-group"> <input type="submit" value="Save" class="btn btn-default" /> </div> </form> </div> </div> <div> <a asp-action="Index">Back to List</a> </div>
نتیجه
در این مقاله نگاهی به روند ساخت یک اپلیکیشن ساده در MVC Core با استفاده از MongoDB به عنوان پایگاه داده کردیم.
شما می توانید source code برای Asp.NetCoreMVCMongoDBDemo را از GitHub دانلود کنید.
- Asp.Net Core
- 1k بازدید
- 3 تشکر