Load شدن تصویر مورد نظر از URL با استفاده از http دراندروید
دوشنبه 21 دی 1394در این مقاله قصد داریم یک عکسی را از یک سایت مورد نظر داخل یک صفحه ی گوشی نمایش دهیم، در این مقاله ما به صورت مستقیم به imageview مورد نظر تصویر را نمایش نمی دهیم و با استفاده از url آدرس را به imageview می دهیم.
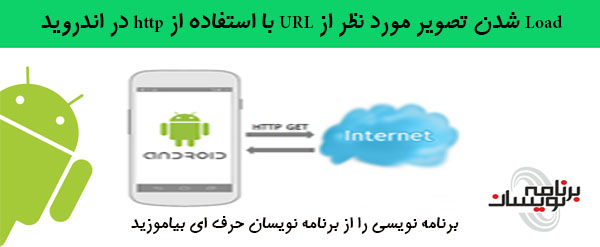
در اینجا شما باید به صورت موقت (cache) عکس را ذخیره نمایید.
یک پروژه ی جدید باز می نماییم، برای بار گذاری تصویر از یک آدرس برای اولین بار نیاز به ذخیره سازی موقت عکس و دریافت آن در imageview مورد نظر داریم، برای ایجاد یک فایل موقت ما نیاز به دسترسی خارجی داریم پس باید داخل فایل androidmanifest مجوزهای دسترسی زیر را بنویسیم:
یکی دسترسی به اینترنت و دیگری دسترسی به ذخیره ساز داخلی :
<uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
در activity مورد نظر قطعه کد زیر را می نویسیم:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Loading image from url" android:layout_margin="10dip" /> <ImageView android:id="@+id/image" android:layout_height="wrap_content" android:layout_width="fill_parent" android:layout_margin="10dip"/> </LinearLayout>
حالا با استفاده از url هر زمان که بخواهید تصویر مورد نظر داخل imageview مورد نظر نمایش داده می شود.
کلاس load تصویر با استفاده از url:
package com.barnamenevisan.loadimage; import android.app.Activity; import android.os.Bundle; import android.widget.ImageView; /** * Created by Esmaili-PC on 05/01/2016. */ public class AndroidLoadImageFromURLActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); // Loader image - will be shown before loading image int loader = R.drawable.loader; // Imageview to show ImageView image = (ImageView) findViewById(R.id.image); // Image url String image_url = "http://api.androidhive.info/images/sample.jpg"; // ImageLoader class instance ImageLoader imgLoader = new ImageLoader(getApplicationContext()); // whenever you want to load an image from url // call DisplayImage function // url - image url to load // loader - loader image, will be displayed before getting image // image - ImageView imgLoader.DisplayImage(image_url, loader, image); } }
در این کلاس imageview مورد نظر را تعریف کرده ایم و url مورد نظر را تعریف می نماییم.و به عکس مورد نظر آدرس مورد نظر را می دهیم.
حالا یک کلاس به نام imageloader بسازید:
package com.barnamenevisan.loadimage; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.InputStream; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; import java.util.Collections; import java.util.Map; import java.util.WeakHashMap; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import android.app.Activity; import android.content.Context; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.widget.ImageView; public class ImageLoader { MemoryCache memoryCache=new MemoryCache(); FileCache fileCache; private Map<ImageView, String> imageViews=Collections.synchronizedMap(new WeakHashMap<ImageView, String>()); ExecutorService executorService; public ImageLoader(Context context){ fileCache=new FileCache(context); executorService=Executors.newFixedThreadPool(5); } int stub_id = R.drawable.ic_launcher; public void DisplayImage(String url, int loader, ImageView imageView) { stub_id = loader; imageViews.put(imageView, url); Bitmap bitmap=memoryCache.get(url); if(bitmap!=null) imageView.setImageBitmap(bitmap); else { queuePhoto(url, imageView); imageView.setImageResource(loader); } } private void queuePhoto(String url, ImageView imageView) { PhotoToLoad p=new PhotoToLoad(url, imageView); executorService.submit(new PhotosLoader(p)); } private Bitmap getBitmap(String url) { File f=fileCache.getFile(url); //from SD cache Bitmap b = decodeFile(f); if(b!=null) return b; //from web try { Bitmap bitmap=null; URL imageUrl = new URL(url); HttpURLConnection conn = (HttpURLConnection)imageUrl.openConnection(); conn.setConnectTimeout(30000); conn.setReadTimeout(30000); conn.setInstanceFollowRedirects(true); InputStream is=conn.getInputStream(); OutputStream os = new FileOutputStream(f); Utils.CopyStream(is, os); os.close(); bitmap = decodeFile(f); return bitmap; } catch (Exception ex){ ex.printStackTrace(); return null; } } //decodes image and scales it to reduce memory consumption private Bitmap decodeFile(File f){ try { //decode image size BitmapFactory.Options o = new BitmapFactory.Options(); o.inJustDecodeBounds = true; BitmapFactory.decodeStream(new FileInputStream(f),null,o); //Find the correct scale value. It should be the power of 2. final int REQUIRED_SIZE=70; int width_tmp=o.outWidth, height_tmp=o.outHeight; int scale=1; while(true){ if(width_tmp/2<REQUIRED_SIZE || height_tmp/2<REQUIRED_SIZE) break; width_tmp/=2; height_tmp/=2; scale*=2; } //decode with inSampleSize BitmapFactory.Options o2 = new BitmapFactory.Options(); o2.inSampleSize=scale; return BitmapFactory.decodeStream(new FileInputStream(f), null, o2); } catch (FileNotFoundException e) {} return null; } //Task for the queue private class PhotoToLoad { public String url; public ImageView imageView; public PhotoToLoad(String u, ImageView i){ url=u; imageView=i; } } class PhotosLoader implements Runnable { PhotoToLoad photoToLoad; PhotosLoader(PhotoToLoad photoToLoad){ this.photoToLoad=photoToLoad; } @Override public void run() { if(imageViewReused(photoToLoad)) return; Bitmap bmp=getBitmap(photoToLoad.url); memoryCache.put(photoToLoad.url, bmp); if(imageViewReused(photoToLoad)) return; BitmapDisplayer bd=new BitmapDisplayer(bmp, photoToLoad); Activity a=(Activity)photoToLoad.imageView.getContext(); a.runOnUiThread(bd); } } boolean imageViewReused(PhotoToLoad photoToLoad){ String tag=imageViews.get(photoToLoad.imageView); if(tag==null || !tag.equals(photoToLoad.url)) return true; return false; } //Used to display bitmap in the UI thread class BitmapDisplayer implements Runnable { Bitmap bitmap; PhotoToLoad photoToLoad; public BitmapDisplayer(Bitmap b, PhotoToLoad p){bitmap=b;photoToLoad=p;} public void run() { if(imageViewReused(photoToLoad)) return; if(bitmap!=null) photoToLoad.imageView.setImageBitmap(bitmap); else photoToLoad.imageView.setImageResource(stub_id); } } public void clearCache() { memoryCache.clear(); fileCache.clear(); } }
در این کلاس عکس مورد نظر load خواهد شد.
حالا کلاس filecache را می نویسیم:
package com.barnamenevisan.loadimage; import java.io.File; import android.content.Context; /** * Created by Esmaili-PC on 05/01/2016. */ public class FileCache { private File cacheDir; public FileCache(Context context){ //Find the dir to save cached images if (android.os.Environment.getExternalStorageState().equals(android.os.Environment.MEDIA_MOUNTED)) cacheDir=new File(android.os.Environment.getExternalStorageDirectory(),"TempImages"); else cacheDir=context.getCacheDir(); if(!cacheDir.exists()) cacheDir.mkdirs(); } public File getFile(String url){ String filename=String.valueOf(url.hashCode()); File f = new File(cacheDir, filename); return f; } public void clear(){ File[] files=cacheDir.listFiles(); if(files==null) return; for(File f:files) f.delete(); } }
یک کلاس برای ذخیره سازی موقت عکس می نویسیم :
package com.barnamenevisan.loadimage; import java.lang.ref.SoftReference; import java.util.Collections; import java.util.HashMap; import java.util.Map; import android.graphics.Bitmap; /** * Created by Esmaili-PC on 05/01/2016. */ public class MemoryCache { private Map<String, SoftReference<Bitmap>> cache=Collections.synchronizedMap(new HashMap<String, SoftReference<Bitmap>>()); public Bitmap get(String id){ if(!cache.containsKey(id)) return null; SoftReference<Bitmap> ref=cache.get(id); return ref.get(); } public void put(String id, Bitmap bitmap){ cache.put(id, new SoftReference<Bitmap>(bitmap)); } public void clear() { cache.clear(); } }
در آخر هم یک کلاس برای دریافت عکس می نویسیم، این کلاس فایل ورودی و خروجی را می گیرد، و به عکس مورد نظر یک اندازه می دهد.
در آخر خروجی کار به صورت زیر خواهد بود:
- Android
- 2k بازدید
- 1 تشکر