دخیره سازی و واکشی تصاویر در بانک اطلاعاتی توسط MVC
یکشنبه 12 دی 1395در این مقاله به شما چگونگی استفاده از یک پایگاه داده برای ذخیره سازی عکس و استفاده از MVC برای فراخوانی این عکس ها با استفاده از شخصی سازی Route ها آموزش میدهیم.
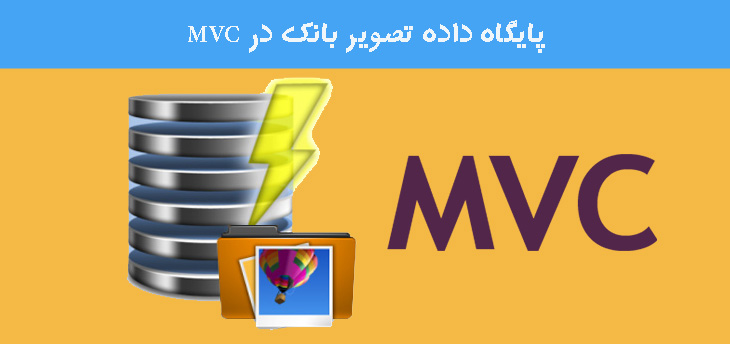
هدف ما ذخیره عکس در پایگاه داده و فراخوانی آن ها در MVC با استفاده از Route ها میباشد.
فرض های ما :
1- URL باید چیزی شبیه به “imagebank/sample-file” یا “imagebank/32403404303“ باشد.
2- کنترلر/اکشن MVC یک عکس از طریق یک ID “sample-file” یا “32403404303” میگیرد و آن ها را از پایگاه داده پیدا میکند و نشان میدهد.
اگر در CACHE موجود بود ، آن را از cache می آورد و در غیر این صورت تصویر را از پایگاه داده می آورد.
پس ما میتوانیم در html به صورت زیر ان را فراخوانی کنیم.
<img src="~/imagebank/sample-file" />
3-اگر شما میخواهید از URL دیگری استفاده کنید شما میتوانید Route بانک عکس خود را در web.config تغییر دهید.
4- اگر شما نمیخواهید که عکس را نمایش دهید و فقط میخواهید آن را دانلود کنید از “imagebank/sample-file/download“ استفاده کنید.
پیکربندی Route بانک عکس در :
App_Start\RouteConfig.cs
public class RouteConfig { public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); routes.MapRoute( name: "ImageBank", url: GetImageBankRoute() + "/{fileId}/{action}", defaults: new { controller = "ImageBank", action = "Index" } ); routes.MapRoute( name: "Default", url: "{controller}/{action}/{id}", defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional } ); } private static string GetImageBankRoute() { var key = "imagebank:routeName"; var config = ConfigurationManager.AppSettings.AllKeys.Contains(key) ? ConfigurationManager.AppSettings.Get(key) : ""; return config ?? "imagebank"; } }
کنترلر بانک تصویر در :
Controllers\ImageBankController.cs
public class ImageBankController : Controller { public ImageBankController() { Cache = new Cache(); Repository = new Repository(); } public ActionResult Index(string fileId, bool download = false) { var defaultImageNotFound = "pixel.gif"; var defaultImageNotFoundPath = $"~/content/img/{defaultImageNotFound}"; var defaultImageContentType = "image/gif"; var cacheKey = string.Format("imagebankfile_{0}", fileId); Models.ImageFile model = null; if (Cache.NotExists(cacheKey)) { model = Repository.GetFile(fileId); if (model == null) { if (download) { return File(Server.MapPath(defaultImageNotFoundPath), defaultImageContentType, defaultImageNotFound); } return File(Server.MapPath(defaultImageNotFoundPath), defaultImageContentType); } Cache.Insert(cacheKey, "Default", model); } else { model = Cache.Get(cacheKey) as Models.ImageFile; } if (download) { return File(model.Body, model.ContentType, string.Concat(fileId, model.Extension)); } return File(model.Body, model.ContentType); } public ActionResult Download(string fileId) { return Index(fileId, true); } private Repository Repository { get; set; } private Cache Cache { get; set; } }
کد بالا دارای دو اکشن است.
یکی از آنها به نام index عکس را نمایش میدهد
و یکی دیگر از متد های اکشن به نام Download تصویر را از cache یا پایگاه داده دانلود میکند.
پایگاه داده repository در :
Repository.cs
public class Repository { public static Models.ImageFile GetFile(string fileId) { //Just an example, use you own data repository and/or database SqlConnection connection = new SqlConnection(ConfigurationManager.ConnectionStrings["ImageBankDatabase"].ConnectionString); try { connection.Open(); var sql = @"SELECT * FROM dbo.ImageBankFile WHERE FileId = @fileId OR ISNULL(AliasId, FileId) = @fileId"; var command = new SqlCommand(sql, connection); command.Parameters.Add("@fileId", SqlDbType.VarChar).Value = fileId; command.CommandType = CommandType.Text; var ada = new SqlDataAdapter(command); var dts = new DataSet(); ada.Fill(dts); var model = new Models.ImageFile(); model.Extension = dts.Tables[0].Rows[0]["Extension"] as string; model.ContentType = dts.Tables[0].Rows[0]["ContentType"] as string; model.Body = dts.Tables[0].Rows[0]["FileBody"] as byte[]; return model; } catch { } finally { if (connection != null) { connection.Close(); connection.Dispose(); connection = null; } } return null; } }
repository بسیار ساده است . این کد فقط برای ظاهر است. شما میتواند کد های خود را در اینجا پیاده سازی کنید.
برای اطلاعات بیشتر در رایطه با repository اینجا کلیک کنید.
کلاس مدل بانک تصاویر در :
Models\ImageFile.cs
public class ImageFile { public byte[] Body { get; set; } public string ContentType { get; set; } public string Extension { get; set; } }
که این کلاس همانطور که ملاحظه میکنید شامل 3 property است.
که property اول برای ذخیره سازی عکس به صورت باینری
property دوم برای گرفتن محتوای عکس
و در آخر property سوم برای گرفتن پسوند عکس پیاده سازی شده اند.
script ساخت جدول :
USE [ImageBankDatabase] GO /****** Object: Table [dbo].[ImageBankFile] Script Date: 11/16/2016 12:36:56 ******/ SET ANSI_NULLS ON GO SET QUOTED_IDENTIFIER ON GO SET ANSI_PADDING ON GO CREATE TABLE [dbo].[ImageBankFile]( [FileId] [nvarchar](50) NOT NULL, [AliasId] [nvarchar](100) NULL, [FileBody] [varbinary](max) NULL, [Extension] [nvarchar](5) NULL, [ContentType] [nvarchar](50) NULL, CONSTRAINT [PK_ImageBankFile] PRIMARY KEY CLUSTERED ( [FileId] ASC )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY] ) ON [PRIMARY] GO SET ANSI_PADDING OFF GO
کلاس Cache provider در :
Cache.cs
public class Cache { public Cache() { _config = ConfigurationManager.GetSection("system.web/caching/outputCacheSettings") as OutputCacheSettingsSection; } private OutputCacheSettingsSection _config; private OutputCacheProfile GetProfile(string profile) { return !string.IsNullOrEmpty(profile) ? _config.OutputCacheProfiles[profile] : new OutputCacheProfile("default"); } private object GetFromCache(string id) { if (string.IsNullOrEmpty(id)) throw new NullReferenceException("id is null"); if (System.Web.HttpRuntime.Cache != null) { lock (this) { return System.Web.HttpRuntime.Cache[id]; } } return null; } public Cache Insert(string id, string profile, object obj) { if (System.Web.HttpRuntime.Cache != null) { if (string.IsNullOrEmpty(id)) { throw new ArgumentNullException("id", "id is null"); } if (string.IsNullOrEmpty(profile)) { throw new ArgumentNullException("profile", string.Format("profile is null for id {0}", id)); } var objProfile = GetProfile(profile); if (objProfile == null) { throw new NullReferenceException(string.Format("profile is null for id {0} and profile {1}", id, profile)); } lock (this) { System.Web.HttpRuntime.Cache.Insert(id, obj, null, DateTime.Now.AddSeconds(objProfile.Duration), TimeSpan.Zero); } } return this; } public bool NotExists(string id) { return GetFromCache(id) == null; } public Cache Remove(string id) { if (System.Web.HttpRuntime.Cache != null) { lock (this) { System.Web.HttpRuntime.Cache.Remove(id); } } return this; } public object Get(string id) { return GetFromCache(id); } }
آموزش asp.net mvc
- ASP.net MVC
- 2k بازدید
- 6 تشکر